Hi Friends,
This is a small article about editor templates in Asp.net MVC. What are they? Why do we need them and what are the scenarios where these editor templates can be used?. Let's find out.
Editor templates are provided in asp.net MVC to generate a view according to any model and that includes complex models too. Now what are complex models? See that by example itself.
Following is the example of a Simple model:
public class Address
{
public string Street { get; set; }
public string PostalCode { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
and the complex one:
public class Person
{
public string Name { get; set; }
public List<Address> Addresses { get; set; }
}
public class Address
{
public string Street { get; set; }
public string PostalCode { get; set; }
public string City { get; set; }
public string Country { get; set; }
}
Now what is the difference. The second one contains a number of Addresses for a particular person name. Now you need to generate a view which can show all of the addresses for a particular person at the same time. What will be the solution?
You may have an idea and that is surely the most lovable "For Loop". For loops are not always good and if we can avoid them,we should. And here we can avoid that by using editor templates. Let's see the how part below:
Just fill the Person model with some dummy data. It should look like following:
public class Person
{
public Person()
{
Name = "Manvendra";
var Agra= new Address
{
City = "Ag",
Country = "IND",
PostalCode = "282004",
Street = "Balkeshwar"
};
var Lucknow= new Address
{
City = "Lko",
Country = "IND",
PostalCode = "226025",
Street = "Mansarovar"
};
var Dehradun= new Address
{
City = "Ddn",
Country = "IND",
PostalCode = "248001",
Street = "Nehru Colony"
};
Addresses = new List<Address> { Agra, Lucknow, Dehradun};
}
public string Name { get; set; }
public List<Address> Addresses { get; set; }
}
We've got some dummy data now. We need to define our editor template. Follow the steps written below:
1-In "Shared" views create a new folder named "Editor Templates".
2- Create a new view by right clicking the Editor Template folder and keep the name of the view exactly same as the model of which you want to make template of. According to my models (above) I need to repeat addresses, so I will keep the name as "Address.cshtml".
3-Put the following markup in the view(Address.cshtml):
@model MvcEditorTemplates.Models.Address
<div class="myclass">
<h3>
Address</h3>
<p>
@Html.LabelFor(x => x.Street)
@Html.TextBoxFor(x => x.Street)
</p>
<p>
@Html.LabelFor(x => x.PostalCode)
@Html.TextBoxFor(x => x.PostalCode)
</p>
<p>
@Html.LabelFor(x => x.City)
@Html.TextBoxFor(x => x.City)
</p>
<p>
@Html.LabelFor(x => x.Country)
@Html.TextBoxFor(x => x.Country)
</p>
</div>
or make it strongly typed with "Address" model. It's your call.
Now that we've defined our editor template, we need to use that. So in your Index action method(that may vary according to your requirements) pass the person model to your main view.
public ActionResult Index()
{
return View(new Person());
}
In your Index view or wherever you want to show the template, call the editor for for the "addresses" in Person model.
@model MvcEditorTemplates.Models.Person
@{
ViewBag.Title = "Editor Template Demo";
}
<h2>Person</h2>
<p>
@Html.LabelFor(model => model.Name)
@Html.EditorFor(model => model.Name)
</p>
<h2>Person Addresses</h2>
@Html.EditorFor(model => model.Addresses)
What should be the result of the editor template we've worked on . Any guesses? Let's see:
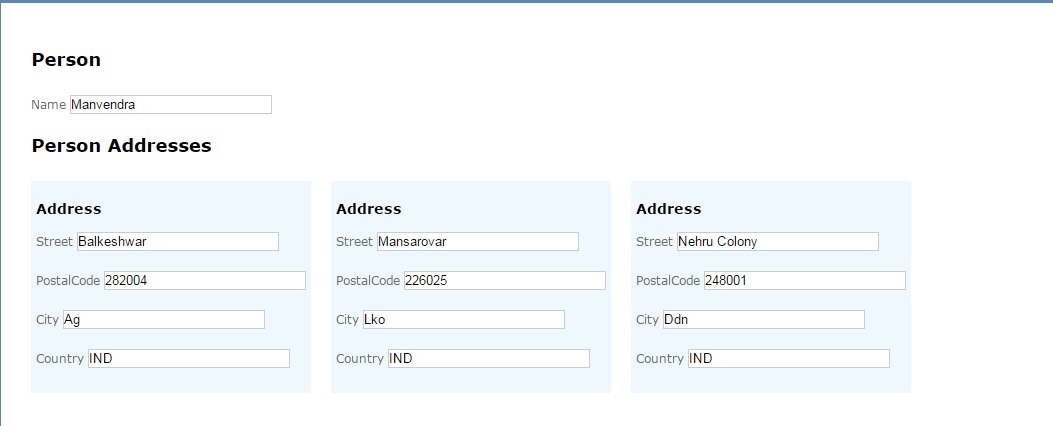
So you can see that we've got multiple addresses for one person and that too without using any for loop by ourselves. Isn't that cool? It can be used as a repeater and possibly multilevel menus. I think it is possible but will post my blog when I am able to do that . Till then..Happy coding.:-)
0 Comment(s)