In this tutorial we will learn to get value from textChange without using any delegate method of UITextField in swift.
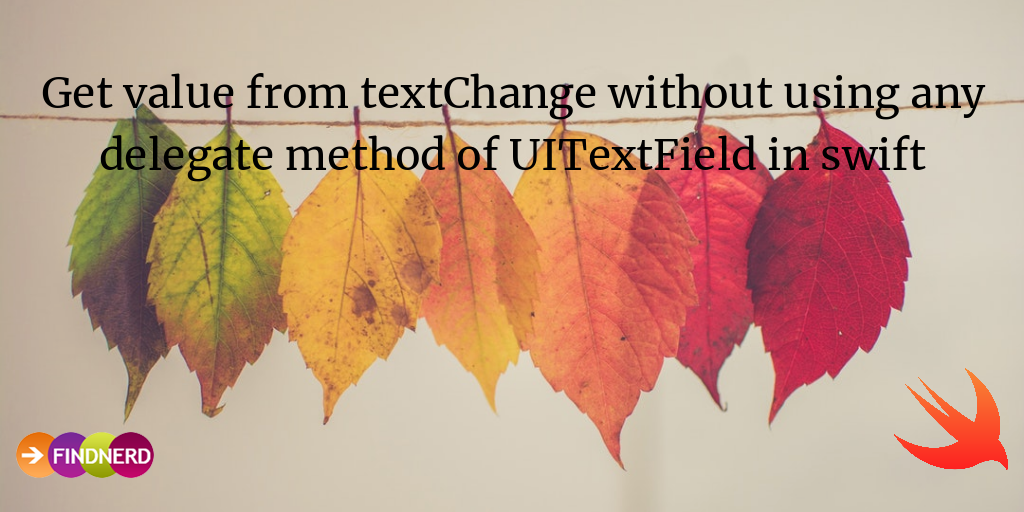
let's see how:
1- We can achieve this using React, i.e. RxSwift
2- No need for textField delegate methods for this.
#Basic setup for project1- Create a new project in Xcode
3- Now we have to install the pods in our project for that jump to project directory.
4- Run the followings commands
a) pod init
b) open podfile
c) Your empty podfile will look like this
# Uncomment the next line to define a global platform for your project
# platform :ios, '9.0'
target 'YourProjectName' do
# Comment the next line if you're not using Swift and don't want to use dynamic frameworks
use_frameworks!
# Pods for YourProjectName
end
Now add these two lines below user_frameworks!
pod 'RxSwift'
pod 'RxCocoa'
now your podfile will look like this
# Uncomment the next line to define a global platform for your project
# platform :ios, '9.0'
target 'YourProjectName' do
# Comment the next line if you're not using Swift and don't want to use dynamic frameworks
use_frameworks!
pod 'RxSwift'
pod 'RxCocoa'
# Pods for YourProjectName
end
d) now run pod install
5- Close your current project and open yourProject.xcworkspace and now we are ready to write code and setup UI.
6- In the main.storyboard add a UITextField and set the autolayout for that according to your need.
7- Also add a UILabel on the UIViewController which will be reprinting the text that we will enter in above textField.
8- Move to viewController.swift file.
import RxSwift
import RxCocoa
@IBOutlet weak var rxTextField:UITextField! //outlet for textFields
@IBOutlet weak var rxLabel:UILabel! //outlet for textFields
let variable = Variable<String>("") //RxSwift String variable
let disposeBag = DisposeBag()
now on viewDidLoad add these lines
_ = rxTextField.rx.text.orEmpty.bind(to:variable).disposed(by:disposeBag)
variable.asObservable().bind(to: rxLabel.rx.text).disposed(by: disposeBag)
or
variable.asObservable().map({ (stringValue) -> String in
return stringValue.uppercased()//will print text in upper case letter in UILabel
}) .bind(to: rxLabel.rx.text).disposed(by: disposeBag)
9- Run your project.
10- As you start typing in textField text will start appear in label.
Your final snapshot for the controller will look like this
import UIKit
import RxSwift
import RxCocoa
class ViewController: UIViewController {
@IBOutlet weak var rxTextField:UITextField!
@IBOutlet weak var rxLabel:UILabel!
let variable = Variable<String>("")
let disposeBag = DisposeBag()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
_ = rxTextField.rx.text.orEmpty.bind(to:variable).disposed(by:disposeBag)
// it will bind the value of textField with the variable.
variable.asObservable().map({ (stringValue) -> String in
return stringValue.uppercased()
}) .bind(to: rxLabel.rx.text).disposed(by: disposeBag)
//as value of variable will change it will start observing that values and start printing that values in the label
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
}
0 Comment(s)