Input tag has many type for the values.
for e.g input type "button" defines the input like button.
In the same way input type "file" defines a box to upload a file to drive.
<input type="file" name="upload"> |
The above code will display a browse button by which user can select a file to upload.
After selecting the file, the file name will appear near the browse button.
But in this we can only select 1 file to upload.
Now the question arises that can we upload multiple files to the drive.
Below is the code to select multiple files to upload:-
<input type="file" id="file" multiple/> |
When multiple is specified in the input, it means user can upload more than one file.
Below is the code to save the uploaded file in a particular folder:-
<html>
<head>
<title>Multiple Upload</title>
</head>
<body>
<!-- the enctype value multipart/form-data is used when uploading file -->
<form method="post" enctype="multipart/form-data">
<input type="file" name="my_file[]" multiple>
<input type="submit" value="Upload">
</form>
<?php
// it will check if the file is selected and count the number of files
if (isset($_FILES['my_file'])) {
$myFile = $_FILES['my_file'];
$fileCount = count($myFile["name"]);
// this loop will run equal to the file count and save the uploaded file in uploads folder
for ($i = 0; $i < $fileCount; $i++) {
if (move_uploaded_file($myFile["tmp_name"][$i] , 'uploads/'.time().$myFile["name"][$i])) {
echo "The file ". basename($myFile["name"][$i] ). " has been uploaded.<BR/>";
}?>
<?php
}
}
?>
</body>
</html>
After running this code, below screen will be open:-
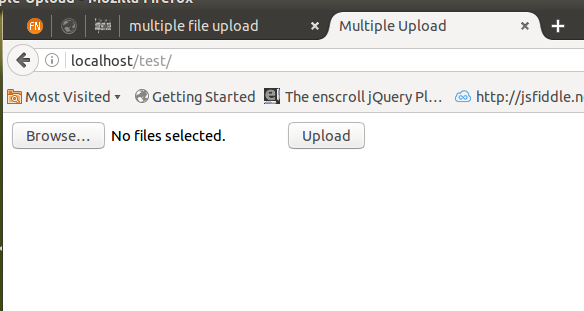
After clicking on the browse button,this window will be opened:-
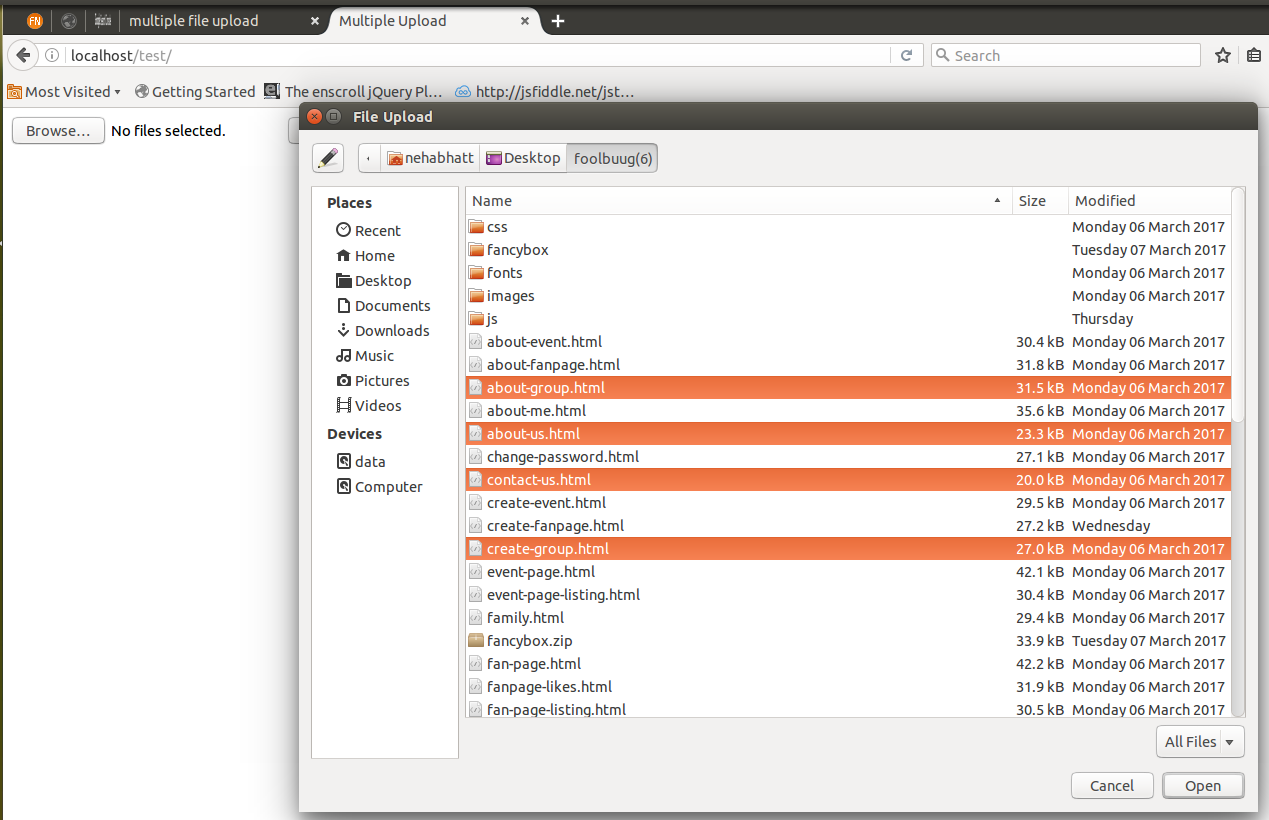
We can selected multiple files from the folder.
After clicking on the upload button a message will be displayed with file name.
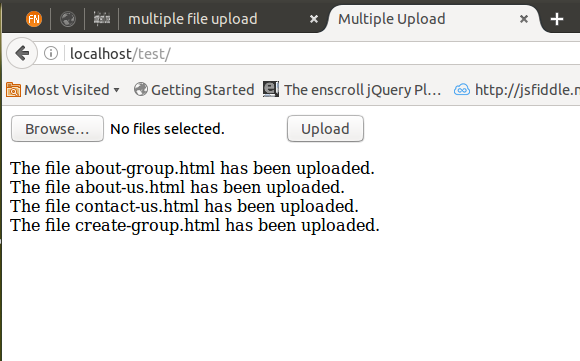
And if we check the "uploads" folder, the selected files will saved there.
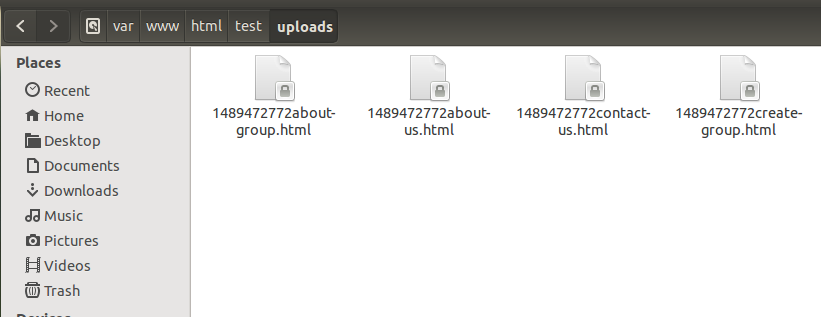
0 Comment(s)