In this post, you will learn how to show mobile ads in your screens. Cordova provides a plugin for google admob. Using this plugin you can show mobile Ad with a line of JavaScript code. It is designed for use in HTML5 based hybrid applications or mobile games.
It supports the following Ad types:
- Banner
- Interstitial (text, picture, video)
- Reward Video
- IAP Ad
- Native Ad (Google new product, on roadmap)
Installing the plugin:
cordova plugin add cordova-plugin-admobpro
For using this plugin in android, you need to download some extras android sdk:
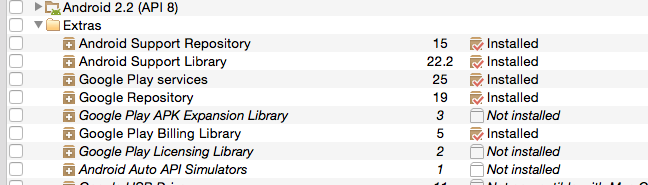
Using the plugin:
To use this plugin, first you need to create Ad Unit Id for your banner and interstitial, in AdMob portal. Then this id will be used in your javascript code.
// select the right Ad Id according to platform
var admobid = {};
if( /(android)/i.test(navigator.userAgent) ) { // for android & amazon-fireos
admobid = {
banner: 'ca-app-pub-xxx/xxx', // or DFP format "/6253334/dfp_example_ad"
interstitial: 'ca-app-pub-xxx/yyy'
};
} else if(/(ipod|iphone|ipad)/i.test(navigator.userAgent)) { // for ios
admobid = {
banner: 'ca-app-pub-xxx/zzz', // or DFP format "/6253334/dfp_example_ad"
interstitial: 'ca-app-pub-xxx/kkk'
};
} else { // for windows phone
admobid = {
banner: 'ca-app-pub-xxx/zzz', // or DFP format "/6253334/dfp_example_ad"
interstitial: 'ca-app-pub-xxx/kkk'
};
}
Methods for banner:
AdMob.createBanner(adId/options, success, fail): To create a banner Ad.
// it will display smart banner at top center, using the default options
AdMob.createBanner({
adId: admobid.banner,
position: AdMob.AD_POSITION.BOTTOM_CENTER,
autoShow: true,
success: function(){
},
error: function(){
alert('failed to create banner');
}
});
AdMob.showBanner(position): To show banner Ad at a position.
AdMob.hideBanner(): It will hide the banner and remove it from screen but you can use it later when needed.
AdMob.removeBanner(): It will destroy the banner, you can not use the banner after calling this method.
Methods for Interstitial:
AdMob.prepareInterstitial(adId/options, success, fail): It will prepare Interstitial add for showing.
// preppare and load ad resource in background, e.g. at begining of game level
if(AdMob) AdMob.prepareInterstitial( {adId:admobid.interstitial, autoShow:false} );
// show the interstitial later, e.g. at end of game level
if(AdMob) AdMob.showInterstitial();
Hope, this will help you to create mobile ads in your application. :)
0 Comment(s)