Hey there!
Form Validation is a very important aspect when it comes to filling forms. It helps us to identify if we are entering the correct information or not. This blog post will particularly deal with the form validation of date and time. I am doing this with javascript. There is pre-specified format mentioned for date and time. For the date fields will only accept input that matches the pattern 'dd/mm/yyyy’ and the time field will allow input starting with : ‘HH:MM’ by an optional 'am' or 'pm'. Anything not entered in this format will not be accepted in the field. A warning message saying “Invalid Format” will be shown on the screen.
So how do we validate the correct input data?
Here is the sample code for this.
HTML:
The code given below will create the form which defines the label and input field inside it. Inside the input field, we will define the placeholder showing the format in which the data has to be entered.
<form onsubmit="checkForm(this); return false;" action="/javascript/validate-date/" method="POST">
<fieldset>
<legend>form Details</legend>
<div class="frm1">
<label for="field_startdate">Start Date</label>
<span>
<input id="field_startdate" type="text" name="startdate" placeholder="dd/mm/yyyy" size="12" >
<small>(dd/mm/yyyy)</small>
</span>
</div>
<div class="frm1">
<label for="field_starttime">Start Time</label>
<span>
<input id="field_starttime" type="text" name="starttime" size="12" placeholder="06:00pm">
<small>(eg. 18:59 or 6:59pm)</small>
</span>
</div>
<span class="submit">
<input type="submit">
</span>
</fieldset>
</form>
CSS:
There CSS given below is a general CSS. It makes the form layout with much styling.
<style type="text/css">
form{
background: green;
color: #fff;
}
.frm1{
margin-bottom: 20px;
}
.submit{
padding-left: 100px;
}
</style>
JavaScript:
Here, in the code given below, a check is made depicting if the input field (date, and then time) is blank or not. If some value has been entered, the entered input is compared to the specified regular format expression. The entered fields accept a pre-defined class \d which represents any numeric character 0-9.
Ex: the regular expression to match a date could also be written as:
re = /^[0-3]?[0-9]\/[01]?[0-9]\/[12][90][0-9][0-9]$/
If the anything is not entered or the input doesn't match the regular expression then warning message will be shown on the screen. The routine stops the form from submitting by returning a false value and the focus is moved to the relevant form field.
If all tests are passed, then a value of true is returned which enables the form to be submitted.
<script type="text/javascript">
function checkForm(form)
{
// regular expression to match required date format
re = /^(\d{1,2})\/(\d{1,2})\/(\d{4})$/;
if(form.startdate.value != '') {
if(regs = form.startdate.value.match(re)) {
// day value between 1 and 31
if(regs[1] < 1 || regs[1] > 31) {
alert("Invalid value for day: " + regs[1]);
form.startdate.focus();
return false;
}
// month value between 1 and 12
if(regs[2] < 1 || regs[2] > 12) {
alert("Invalid value for month: " + regs[2]);
form.startdate.focus();
return false;
}
// year value between 1902 and 2016
if(regs[3] < 1902 || regs[3] > (new Date()).getFullYear()) {
alert("Invalid value for year: " + regs[3] + " - must be between 1902 and " + (new Date()).getFullYear());
form.startdate.focus();
return false;
}
} else {
alert("Invalid date format: " + form.startdate.value);
form.startdate.focus();
return false;
}
}
// regular expression to match required time format
re = /^(\d{1,2}):(\d{2})([ap]m)?$/;
if(form.starttime.value != '') {
if(regs = form.starttime.value.match(re)) {
if(regs[3]) {
// 12-hour value between 1 and 12
if(regs[1] < 1 || regs[1] > 12) {
alert("Invalid value for hours: " + regs[1]);
form.starttime.focus();
return false;
}
} else {
// 24-hour value between 0 and 23
if(regs[1] > 23) {
alert("Invalid value for hours: " + regs[1]);
form.starttime.focus();
return false;
}
}
// minute value between 0 and 59
if(regs[2] > 59) {
alert("Invalid value for minutes: " + regs[2]);
form.starttime.focus();
return false;
}
} else {
alert("Invalid time format: " + form.starttime.value);
form.starttime.focus();
return false;
}
}
alert("All input fields have been validated!");
return true;
}
</script>
Output:
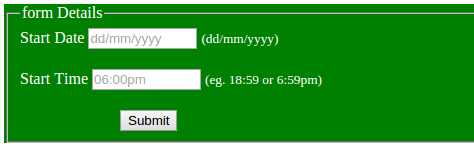
0 Comment(s)