Unlike in website the session are useful for handling users data from one page to another, The API requires a secret token to do the same.
The secret information like email, user type, balance need to be get passed from one request to another in API via tokens.
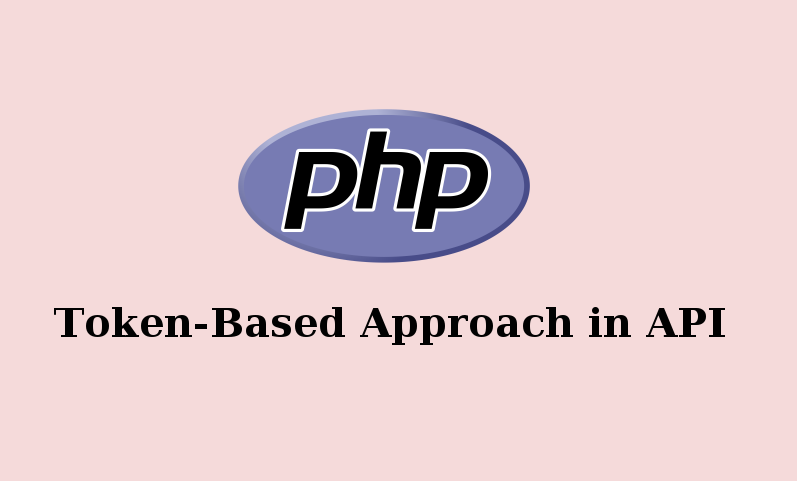
In this tutorial, we will see, how to create token based approach to make an authentic requests on API.
- So first, let's start building an example where we will pass user info from one API hit to another hit
$requestHeaders = apache_request_headers();
if (isset($requestHeaders['Authorization']) && !empty($requestHeaders['Authorization']))
{
$authorizationHeader = $requestHeaders['Authorization'];
if (!$this->_authenticate($authorizationHeader))
{
header('Content-Type: application/json');
exit(json_encode(array(
'status' => 'failure',
'message' => 'No valid access token provided.'
)));
}
else
{
$this->$action();
}
}
else
{
header('Content-Type: application/json');
exit(json_encode(array(
'status' => 'failure',
'message' => 'No authorization header sent.'
)));
}
In the above PHP code we are using Authorization Header, This is the token which we will validate and use for getting the user's info.
- Now will verify this request and it's code goes like this:-
protected
function _authenticate($AccesToken)
{
return $this->_validateToken($AccesToken);
}
/**
* This function validate token send by user.
*
* @access protected
* @param string $AccesToken
* @return bool true|false
*/
protected
function _validateToken($AccesToken)
{
$this->db->select('id, username,mobile, email, device_type, device_token, first_name, last_name, modified');
$this->db->where('access_token', $AccesToken);
$this->db->where('delete', '0');
$this->db->from('users');
$query = $this->db->get();
$find_user = $query->row_array();
if (count($find_user) > 0)
{
$data['UserId'] = $find_user['id'];
$data['Username'] = $find_user['username'];
$data['FirstName'] = $find_user['first_name'];
$data['LastName'] = $find_user['last_name'];
$data['Mobile'] = $find_user['mobile'];
$data['Email'] = $find_user['email'];
$data['DeviceType'] = $find_user['device_type'];
$data['DeviceToken'] = $find_user['device_token'];
$data['Modified'] = $find_user['modified'];
$this->current_user = $data;
return true;
}
return false;
}
In the code above you can see the select query is validating and fetching the user details for i.e- FirstName, LastName, Email, Mobile etc. Once this token is found match with any user's detail, then these details will be set on the public var current_user.
In the case if token does'nt found matched with any user, web server will return a false message in JSON form.
- Now you have to call this function from every API and by doing using the below method:
$this->_validateToken($AccesToken);
The above method will authenticate and fetch the users detail into a variable. that variable can be used as a public which you can be accessed in another API.
So, this how we makes a token based approach which do same function as sessions do in website. If you have any questions or inputs plese feel free to write in comment section below.
0 Comment(s)