Many times the user forget their password which they opt at the time of registration, and the developer who is new in Codeigniter framework struggles to create this module. So, in this tutorial, I will guide you to make the forgot password module in Codeigniter.
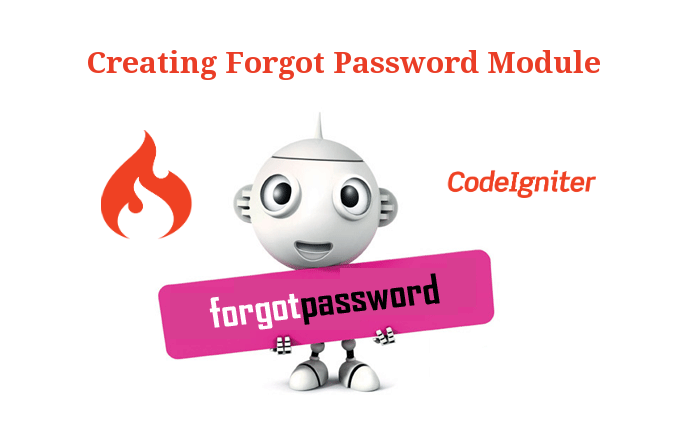
Now, lets start building this:-
As the developer point of view every step is to be consider in coding. So all the cases must be ended in a pre defined manner. Like if email is not found or password is not sent. The first part is the html which will go like this:-
<div class="modal fade" id="myModal" tabindex="-1" role="dialog" aria-labelledby="myModalLabel">
<div class="modal-dialog" role="document">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-label="Close"><span aria-hidden="true">×</span></button>
<h4 class="modal-title" id="myModalLabel">Reset your password</h4>
</div>
<div class="modal-body">
<form id="resetPassword" name="resetPassword" method="post" action="<?php echo base_url();?>user/ForgotPassword" onsubmit ='return validate()'>
<table class="table table-bordered table-hover table-striped">
<tbody>
<tr>
<td>Enter Email: </td>
<td>
<input type="email" name="email" id="email" style="width:250px" required>
</td>
<td><input type = "submit" value="submit" class="button"></td>
</tr>
</tbody> </table></form>
<div id="fade" class="black_overlay"></div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-default" data-dismiss="modal">Close</button>
</div>
</div>
</div>
</div>
Now to handle this request you have to write the code in your controller which will go like this:-
/*
Forgotpassword email sender:
No view
*/
public function ForgotPassword()
{
$email = $this->input->post('email');
$findemail = $this->usermodel->ForgotPassword($email);
if($findemail){
$this->usermodel->sendpassword($findemail);
}else{
$this->session->set_flashdata('msg',' Email not found!');
redirect(base_url().'user/Login','refresh');
}
}
Now put the mysql code for fetching the matching email from database, If email is not found then it will alert user that email is incorrect but if email is found then function will send the new password to current email id. And it's code will go like this:-
//funtion to get email of user to send password
public function ForgotPassword($email)
{
$this->db->select('email');
$this->db->from('user_registrations');
$this->db->where('email', $email);
$query=$this->db->get();
return $query->row_array();
}
public function sendpassword($data)
{
$email = $data['email'];
$query1=$this->db->query("SELECT * from user_registrations where email = '".$email."' ");
$row=$query1->result_array();
if ($query1->num_rows()>0)
{
$passwordplain = "";
$passwordplain = rand(999999999,9999999999);
$newpass['password'] = md5($passwordplain);
$this->db->where('email', $email);
$this->db->update('user_registrations', $newpass);
$mail_message='Dear '.$row[0]['first_name'].','. "\r\n";
$mail_message.='Thanks for contacting regarding to forgot password,<br> Your <b>Password</b> is <b>'.$passwordplain.'</b>'."\r\n";
$mail_message.='<br>Please Update your password.';
$mail_message.='<br>Thanks & Regards';
$mail_message.='<br>Your company name';
date_default_timezone_set('Etc/UTC');
require FCPATH.'assets/PHPMailer/PHPMailerAutoload.php';
$mail = new PHPMailer;
$mail->isSMTP();
$mail->SMTPSecure = "tls";
$mail->Debugoutput = 'html';
$mail->Host = "yooursmtp";
$mail->Port = 587;
$mail->SMTPAuth = true;
$mail->Username = "your@email.com";
$mail->Password = "password";
$mail->setFrom('admin@site', 'admin');
$mail->IsHTML(true);
$mail->addAddress($email);
$mail->Subject = 'OTP from company';
$mail->Body = $mail_message;
$mail->AltBody = $mail_message;
if (!$mail->send()) {
$this->session->set_flashdata('msg','Failed to send password, please try again!');
} else {
$this->session->set_flashdata('msg','Password sent to your email!');
}
redirect(base_url().'user/Login','refresh');
}
else
{
$this->session->set_flashdata('msg','Email not found try again!');
redirect(base_url().'user/Login','refresh');
}
}
And the output will be appear like this in the screenshot as below:-
2 Comment(s)