Hello friends, today I am going to give basic understanding of installing Swagger in Cakephp 3. Before Installing it, let us understand what is Swagger. Swagger is the most powerful framework which is designed to develop APIS and to create documentation. Just like postman, you can also test APIS by using Swagger. Lets start installing it.
Installing Swagger in Cakephp 3.3
Here we are going to use Cakephp 3 plugin which will going to add auto generated documentation in Swagger 2.0 using swagger-php and swagger-ui. Follow the steps below:
Step 1: We need to install the plugin first using composer:
composer require alt3/cakephp-swagger:dev-master
This command will generate the following output:
Loading composer repositories with package information
Updating dependencies (including require-dev)
Package operations: 5 installs, 0 updates, 0 removals
- Installing symfony/finder (v3.2.1) Downloading: 100%
- Installing doctrine/lexer (v1.0.1) Downloading: 100%
- Installing doctrine/annotations (v1.2.7) Downloading: 100%
- Installing zircote/swagger-php (2.0.8) Downloading: 100%
- Installing alt3/cakephp-swagger (dev-master b4b21a6) Cloning b4b21a6f35
Writing lock file
Generating autoload files
> Cake\Composer\Installer\PluginInstaller::postAutoloadDump
Step 2: Now either run the following command in order to enable the plugin:
bin/cake plugin load Alt3/Swagger --routes
This command will generate the following:
Welcome to CakePHP v3.3.11 Console
---------------------------------------------------------------
App : src
Path: /var/www/bikewale/src/
PHP : 5.5.9-1ubuntu4.20
---------------------------------------------------------------
/var/www/bikewale/config/bootstrap.php modified
or you can also add the following line manually to your config/bootstrap.php
file:
Plugin::load('Alt3/Swagger', ['routes' => true]);
Step 3: Now browse the following URL: http://localhost/bikewale/alt3/swagger . This page will now produce the Swagger-UI interface:
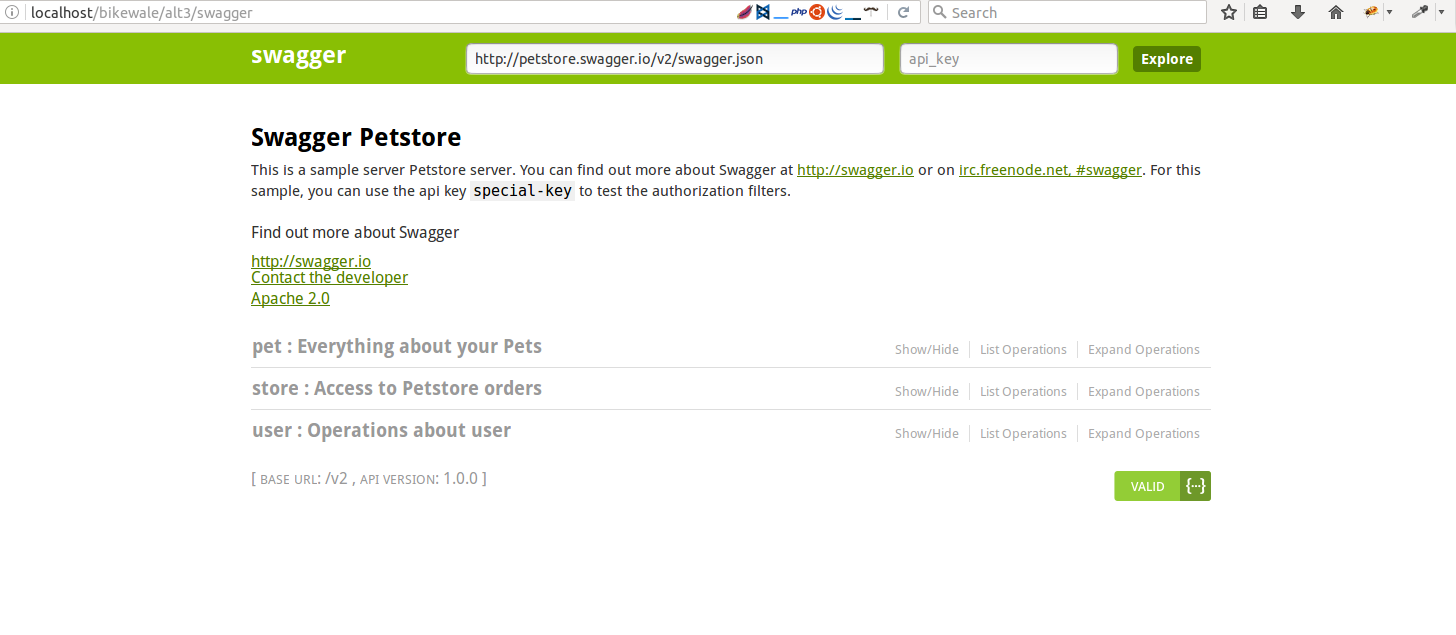
Configuring Swagger
Following file /config/swagger.php will be used to configure swagger plugin. Example is shown below:
<?php
use Cake\Core\Configure;
return [
'Swagger' => [
'ui' => [
'title' => 'ALT3 Swagger',
'validator' => true,
'api_selector' => true,
'route' => '/swagger/',
'schemes' => ['http', 'https']
],
'docs' => [
'crawl' => Configure::read('debug'),
'route' => '/swagger/docs/',
'cors' => [
'Access-Control-Allow-Origin' => '*',
'Access-Control-Allow-Methods' => 'GET, POST',
'Access-Control-Allow-Headers' => 'X-Requested-With'
]
],
'library' => [
'api' => [
'include' => ROOT . DS . 'src',
'exclude' => [
'/Editor/'
]
],
'editor' => [
'include' => [
ROOT . DS . 'src' . DS . 'Controller' . DS . 'AppController.php',
ROOT . DS . 'src' . DS . 'Controller' . DS . 'Editor',
ROOT . DS . 'src' . DS . 'Model'
]
]
]
]
];
User Interface
Following Swagger-UI options can be used to customize the user interface of swagger:
i) title: This will set the title of the browser to cakephp-swagger which is default.
ii) validator: You can hide or show the validator image. Default value is true.
iii) route: default routing is set to /alt3/swagger/. You can change it.
iv) schemes: This is used to generate base url. Default is null
'library' => [
'api' => [
'include' => ROOT . DS . 'src',
'exclude' => [
'/Editor/'
]
],
'editor' => [
'include' => [
ROOT . DS . 'src' . DS . 'Controller' . DS . 'AppController.php',
ROOT . DS . 'src' . DS . 'Controller' . DS . 'Editor',
ROOT . DS . 'src' . DS . 'Model'
]
]
]
You can browse the following url: http://localhost/bikewale/alt3/swagger/docs which will produce a json list with links . Example is shown below:
{
"success": true,
"data": [
{
"document": "api",
"link": "http://your.app/alt3/swagger/docs/api"
},
{
"document": "editor",
"link": "http://your.app/alt3/swagger/docs/editor"
}
]
}
Copy and paste the following code to controller in cakephp
<?php
/**
@SWG\Swagger(
@SWG\Info(
title="cakephp-swagger",
description="Quickstart annotation example",
termsOfService="http://swagger.io/terms/",
version="1.0.0"
)
)
@SWG\Get(
path="/cakephp3/api/v1.0/users",
summary="Retrieve a list of users",
tags={"user"},
consumes={"application/json"},
produces={"application/xml", "application/json"},
@SWG\Parameter(
name="sort",
description="Sort results by field",
in="query",
required=false,
type="string",
enum={"name", "description"}
),
@SWG\Response(
response="200",
description="Successful operation",
@SWG\Schema(
type="array",
ref="#/definitions/User"
)
),
@SWG\Response(
response=429,
description="Rate Limit Exceeded"
)
)
@SWG\Definition(
definition="User",
required={"name", "description"},
@SWG\Property(
property="id",
type="integer",
description="CakePHP record id"
),
@SWG\Property(
property="name",
type="string",
description="CakePHP name field"
),
@SWG\Property(
property="description",
type="string",
description="Description of a most tasty cocktail"
)
)
*/
/**
@SWG\Post(
path="/cakephp3/api/v1.0/createusers",
summary="Add user",
tags={"user"},
consumes={"application/json"},
produces={"application/json"},
@SWG\Parameter(
name="first_name",
description="User's first name",
in="formData",
required=true,
type="string"
),
@SWG\Parameter(
name="last_name",
in="formData",
description="User's last name",
required=true,
type="string"
),
@SWG\Parameter(
name="email",
in="formData",
description="User's email",
required=true,
type="string",
format="email"
),
@SWG\Parameter(
name="password",
in="formData",
description="User's password",
required=true,
type="string",
format="password"
),
@SWG\Parameter(
name="role",
description="User's role",
in="formData",
required=true,
type="string",
enum={"client", "consultant", "admin"}
),
@SWG\Parameter(
name="profile_pic",
description="User's pic",
in="formData",
required=false,
type="file"
),
@SWG\Parameter(
name="duration",
in="formData",
description="User's duration",
required=true,
type="integer"
),
@SWG\Response(
response="200",
description="Successful operation",
@SWG\Schema(
type="array",
ref="#/definitions/User"
)
),
@SWG\Response(
response=429,
description="Rate Limit Exceeded"
)
)
@SWG\Definition(
definition="Create User",
required={"name", "description"},
@SWG\Property(
property="id",
type="integer",
description="CakePHP record id"
),
@SWG\Property(
property="name",
type="string",
description="CakePHP name field"
),
@SWG\Property(
property="description",
type="string",
description="Description of a most tasty cocktail"
)
)
*/
This would result in following:
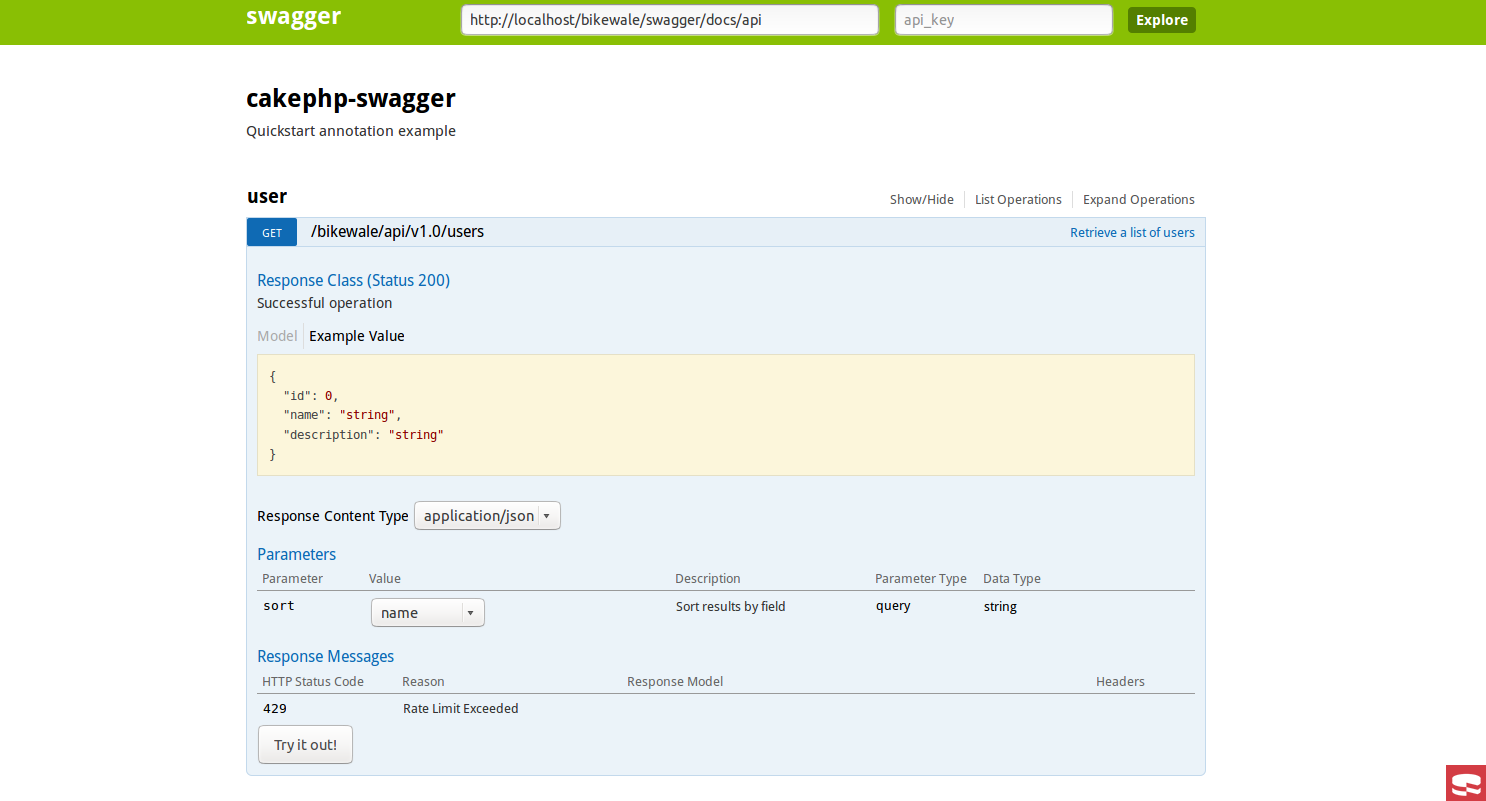
Hope this blog will help to install swagger in cakephp 3.x .
Thanks for reading the blog.
0 Comment(s)