"Returning a file to View/Download in MVC"
In this article I will try to explain how to view and download a file in Asp.Net MVC Application.
Let us understand it with the help of the folowing example.
Getting Started:
Step 1: Create a Asp.Net MVC Application.
Step 2: Create a Controller inside the controller folder (say: "SampleController"). Then the controller will look like:
using FileDownload.Models;
using FileDownload.Service;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace FileDownload.Controllers
{
public class SampleController : Controller
{
public ActionResult Index()
{
return View();
}
}
}
Step 3: Create a model (say: "DownLoadFileInformation") as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace FileDownload.Models
{
public class DownLoadFileInformation
{
public int FileId { get; set; }
public string FileName { get; set; }
public string FilePath { get; set; }
}
}
Step 4: Create a folder (say:"MyFiles") inside the Application. This will create the files to be displayed and downloaded. In my case as the application is stored in c drive therefore the location of the folder is "C:\Users\dotnet1\Desktop\POC\FileDownload\FileDownload\MyFiles" where FileDownload is the name of the Application.
Step 5: Now create a class (say: "DownloadFiles") inside a folder (say:"Service") and write the following code:
using FileDownload.Models;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Web;
using System.Web.Hosting;
namespace FileDownload.Service
{
public class DownloadFiles
{
public List<DownLoadFileInformation> GetFiles()
{
List<DownLoadFileInformation> lstFiles = new List<DownLoadFileInformation>();
DirectoryInfo dirInfo = new DirectoryInfo(HostingEnvironment.MapPath("~/MyFiles"));
int i = 0;
foreach (var item in dirInfo.GetFiles())
{
lstFiles.Add(new DownLoadFileInformation()
{
FileId = i + 1,
FileName = item.Name,
FilePath = dirInfo.FullName + @"\" + item.Name
});
i = i + 1;
}
return lstFiles;
}
}
}
This class file contains the method "GetFiles()" which will get all the files from the "MyFiles" folder and bind it to the list of model type.
Step 6: Now go back to the controller and write the following code:
using FileDownload.Models;
using FileDownload.Service;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace FileDownload.Controllers
{
public class SampleController : Controller
{
DownloadFiles obj;
public SampleController()
{
obj = new DownloadFiles();
}
public ActionResult Index()
{
var filesCollection = obj.GetFiles();
return View(filesCollection);
}
public FileResult Download(string FileID)
{
int CurrentFileID = Convert.ToInt32(FileID);
var filesCol = obj.GetFiles();
string CurrentFileName = (from fls in filesCol where fls.FileId == CurrentFileID
select fls.FilePath).First();
string contentType = string.Empty;
if (CurrentFileName.Contains(".txt"))
{
contentType = "application/txt";
}
else if (CurrentFileName.Contains(".docx"))
{
contentType = "application/docx";
}
return File(CurrentFileName, contentType, CurrentFileName);
}
}
}
The method "Download(string FileID)" is responsible for downloading the file. The method obj.GetFiles() will call the method of the DownloadFiles class which will get all the files inside the "MyFiles" folder.
Step 7: Now right click on the Index Action Method and Add View as follows:
@model IEnumerable<FileDownload.Models.DownLoadFileInformation>
<div>
@{
var x = Model;
<ul>
@foreach (var item in x)
{
<li>@item.FileName </li>@Html.ActionLink("Download", "Download", new { FileID =
item.FileId })
}
</ul>
}
</div>
In this all the files from the "MyFiles" folder first will be listed along with the corresponding Download Link. On click of the download link the corresponding file gets downloaded.
Output:
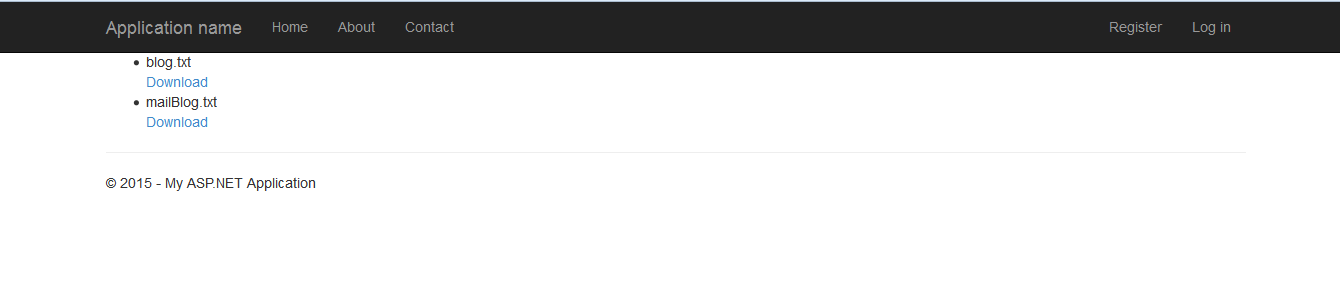
Onclicking the Download link the corresponding file gets downloaded.
Hope it helps...Happy Coding!
0 Comment(s)