"Partial View in Asp.Net MVC"
Key Features:
1. The View defined inside the parent View is called the Partial View.
2. It is same like a user control (.ascx) in Asp.Net.
3. The Partial View has access to the data of the parent View.
4. The changes made by the Partial View updates only partial view's ViewData object, those updates do not affect the Parent View's data.
5. By using Partial View we can create a reusable content in the project.
Let us understand it more clearly with the help of an Example:
In this example we will render a Partial View (PartialIndex.cshtml) inside a Parent View (Index.cshtml).We will also create a List of type PartialView (Model Class) and pass it to the Partial View from the Parent View and that List will be iterated and displayed inside the Partial View.
Step 1: Create a Asp.Net MVC Application.
Step 2: Now Create a Model (say "PartialView") inside the Models Folder, as follows:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace MultipleSubmit.Models
{
public class PartialView
{
public string name {get; set;}
public string address { get; set; }
public List<PartialView> partialModel { get; set; }
}
}
In this code we have two properties name and address and a List of Class ("PartialView") type.
Step 3: Now go to the Controller and write the code as follows:
using MultipleSubmit.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MultipleSubmit.Controllers
{
public class MultipleSubmitController : Controller
{
public ActionResult Index()
{
return View(new PartialView { partialModel = Sampledetails() });
}
private List<PartialView> Sampledetails()
{
List<PartialView> model = new List<PartialView>();
model.Add(new PartialView()
{
name = "Rima",
address = "Kannur"
});
model.Add(new PartialView()
{
name = "Rohan",
address = "Ernakulam"
});
model.Add(new PartialView()
{
name = "Reshma",
address = "Kannur"
});
return model;
}
}
}
In this code we passed a list of PartialView type to the View Index.cshtml. This List was filled by the method Sampledetails().
Step 4: Now in the Index.cshtml render the Partial View (PartialIndex) as follows:
@model MultipleSubmit.Models.PartialView
@{
ViewBag.Title = "Index";
}
<h2>Index</h2>
<div>
@Html.Partial("~/Views/Home/PartialIndex.cshtml", Model.partialModel)
</div>
In the above code @Html.Partial("~/Views/Home/PartialIndex.cshtml", Model.partialModel) is the line responcible for rendering Partial View.
Step 5: Now we have to create a Partial View, for which Go to the Views then right click on the View folder and select Add >> View, select create Partial View and submit. Partial View will be created.
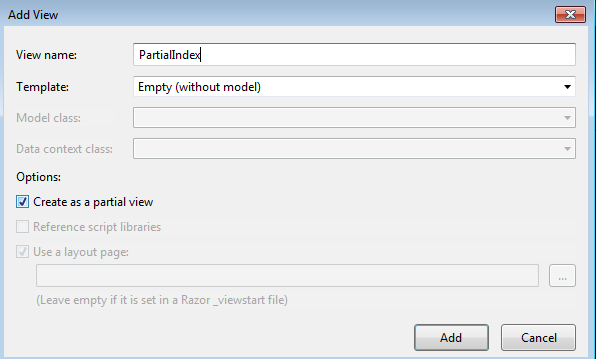
Step 6: Now write the following code in the Partial View:
@model IEnumerable<MultipleSubmit.Models.PartialView>
@if (Model != null)
{
<div class="grid">
<table cellspacing="0" width="80%">
<thead>
<tr>
<th>
Name
</th>
<th>
Address
</th>
</tr>
</thead>
<tbody>
@foreach (var item in Model)
{
<tr>
<td align="left">
@item.name
</td>
<td align="left">
@item.address
</td>
</tr>
}
</tbody>
</table>
</div>
}
Step 7: Now run the application, you will get the otuput as follows:
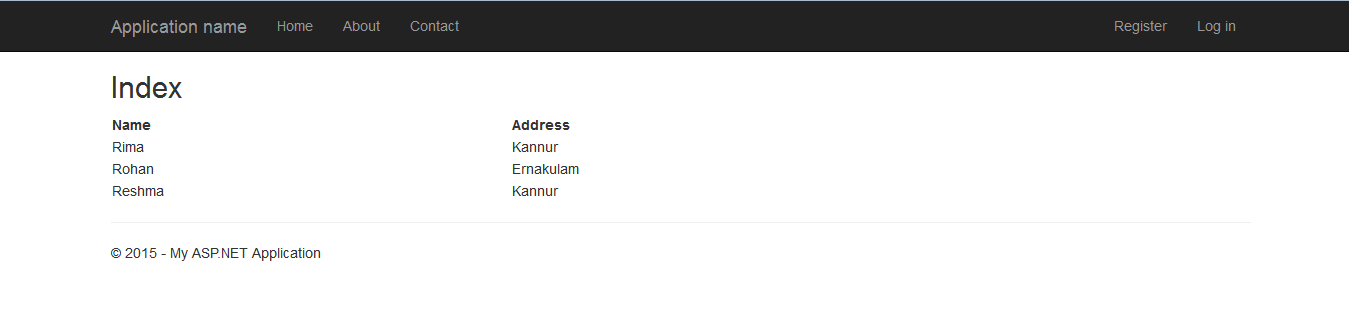
This is how you render Partial View inside Parent View.
Hope it helps...Happy Coding!
0 Comment(s)