In a web application, we often use common section in most of the pages i.e. Menu, Side Navigations, Footer etc. So in order to not write the same code in all the pages, we have the provisions of Layout view in MVC.
Layout view works in the same way as Master pages used to work in ASP.Net application.
It contains different sections for rendering the different repetitive elements.
Following is the common structure of Layout View.
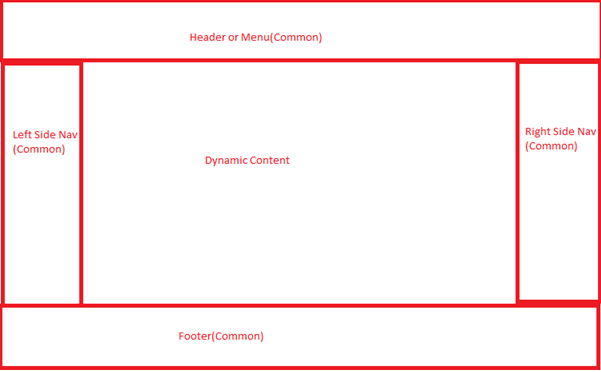
Here in the above document, we have different sections for rendering different type of content. Header section be used for Menu and header related stuff, Footer section may contain footer stuff and Left and Right Nav section may contain the stuff that you want to show in left and right section of the webpage.
Use of Layout View
When we create a MVC application, we get default layout page under the shared folder in view folder. Here is the snapshot of default scaffolding of MVC application
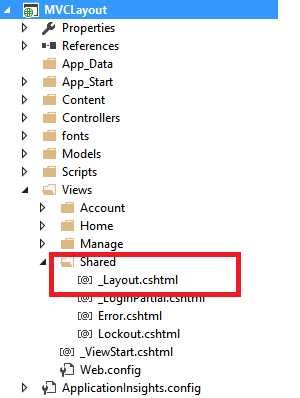
In the above document, we have _Layout.cshtml view which is the default layout view under shared folder. Shared folder inside view folder is used for keeping the common views which are shared across different views in application. It is good practice to store all the Layout Views inside the shared folder.
Default structure of Layout page is as follows
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>@ViewBag.Title - My ASP.NET Application</title>
@Styles.Render("~/Content/css")
@Scripts.Render("~/bundles/modernizr")
</head>
<body>
<div class="navbar navbar-inverse navbar-fixed-top">
<div class="container">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-collapse">
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
@Html.ActionLink("Application name", "Index", "Home", new { area = "" }, new { @class = "navbar-brand" })
</div>
<div class="navbar-collapse collapse">
<ul class="nav navbar-nav">
<li>@Html.ActionLink("Home", "Index", "Home")</li>
<li>@Html.ActionLink("About", "About", "Home")</li>
<li>@Html.ActionLink("Contact", "Contact", "Home")</li>
</ul>
@Html.Partial("_LoginPartial")
</div>
</div>
</div>
<div class="container body-content">
@RenderBody()
<hr />
<footer>
<p>© @DateTime.Now.Year - My ASP.NET Application</p>
</footer>
</div>
@Scripts.Render("~/bundles/jquery")
@Scripts.Render("~/bundles/bootstrap")
@RenderSection("scripts", required: false)
</body>
</html>
It is simple HTML page with some menu items and Footer. The interesting thing here is that it has RenderBody and RenderSection methods
Render body works in the same way as contentplaceholder used to works in ASP.Net. You can render your dynamic view content inside the RenderBody method.
For example, here is the code of Index view inside home folder
Assigning Layout Page to view
@{
Layout = "~/Views/Shared/_Layout.cshtml";
ViewBag.Title = "Home Page";
}
<div class="jumbotron">
<h1>ASP.NET</h1>
<p class="lead">ASP.NET is a free web framework for building great Web sites and Web applications using HTML, CSS and JavaScript.</p>
<p><a href="http://asp.net" class="btn btn-primary btn-lg">Learn more »</a></p>
</div>
<div class="row">
<div class="col-md-4">
<h2>Getting started</h2>
<p>
ASP.NET MVC gives you a powerful, patterns-based way to build dynamic websites that
enables a clean separation of concerns and gives you full control over markup
for enjoyable, agile development.
</p>
<p><a class="btn btn-default" href="http://go.microsoft.com/fwlink/?LinkId=301865">Learn more »</a></p>
</div>
<div class="col-md-4">
<h2>Get more libraries</h2>
<p>NuGet is a free Visual Studio extension that makes it easy to add, remove, and update libraries and tools in Visual Studio projects.</p>
<p><a class="btn btn-default" href="http://go.microsoft.com/fwlink/?LinkId=301866">Learn more »</a></p>
</div>
<div class="col-md-4">
<h2>Web Hosting</h2>
<p>You can easily find a web hosting company that offers the right mix of features and price for your applications.</p>
<p><a class="btn btn-default" href="http://go.microsoft.com/fwlink/?LinkId=301867">Learn more »</a></p>
</div>
</div>
If you will see the above code, you will find that it does not contain any head or body tag of html. But at the top, it defines the layout view for the view
@{
Layout = "~/Views/Shared/_Layout.cshtml";
ViewBag.Title = "Home Page";
}
Above lines suggest the view engine that the index page will be rendered inside the RenderBody method of the _Layout.cshtml page.
So the final output of _LayoutPage merged with Index page will look like this
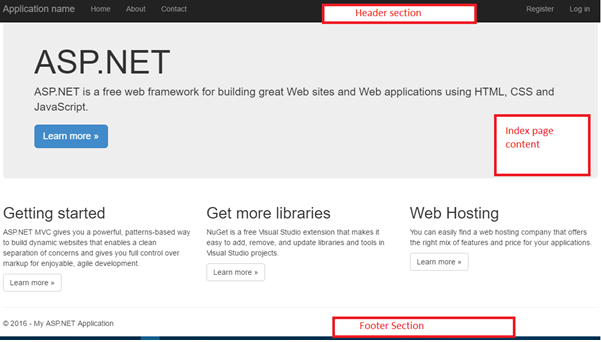
In the above snapshot, Header and footer section are from _Layout view and the content of Index page is render where we had mention RenderBody method in _Layout view.
Different Rendering Methods in Layout
There are different rendering methods in MVC layout view a=which are used for different purposes
Here is the list
1. Styles.Render
2. Scripts.Render
3. RenderSection
4. RenderBody
5. RenderPage
Style.Render
Style.Render is used for rendeting the bundle of css files defined In BundleConfig.cs file. To render styles, first we need to define it in budleconfig.cs file under RegisterBundles method like below
bundles.Add(new StyleBundle("~/Content/css").Include(
"~/Content/bootstrap.css",
"~/Content/site.css"));
Then in the layout page, we write following lines
@Styles.Render("~/Content/css")
Scripts.Render
Scripts.Render is used for rendering scripts files defined in BundleConfig.cs file. To render scripts, first, we need to define it in budleconfig.cs file under RegisterBundles method like below
bundles.Add(new ScriptBundle("~/bundles/jquery").Include(
"~/Scripts/jquery-{version}.js"));
Then in the layout page, we write following lines
@Scripts.Render("~/bundles/jquery")
RenderSection
RenderSection is used for rendering a section of HTML code by its name
Here is the example
@section sideNav{
<h1>Side Nav Content</h1>
}
Here the section name in child view is the header. If we want to render this section in layout page then we will write the following line in layout page
@RenderSection("sideNav")
RenderBody
RenderBody is used for rendering the child page content. The example of renderbody we have already discussed
RenderPage
RenderPage method is used for rendering pages in a layout. A layout page can have multiple RenderPage methods.
@RenderPage("~/Views/Shared/_Header.cshtml")
0 Comment(s)