A dialogue box is called modal when it restricts the other functionality of the page until the dialogue box has been closed.
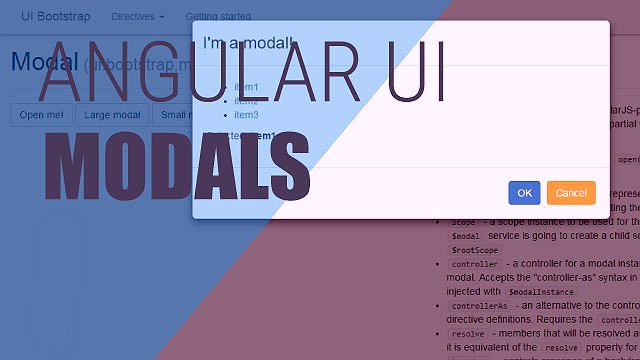
Here is the code for opening a simple modal popup using ‘modals’ in angularjs.
first create a simple HTML template and call a function on a button click in which the modal will get open:
<html>
<div class="maiWidth">
<h2 class=“adminhead”>Admin List</h2>
<div class="col-sm-3 columns">
<button type="" class="btn grn-btn" ng-click=“Confirm()”>Confirm Admin
</button>
</div>
</div>
</html>
In the above code I have called a confirm function on a button click.
Then add this script for the modal template like this without html tag.
<html>
<script type="text/ng-template" id="confirmAlert.html">
<div class="modal-body">
<div class="innerWrap sub-modal">
<p>
Do you want to continue?
</p>
<form class="form-horizontal">
<div class="row btn-col min-btn">
<div class="col-sm-4 col-sm-offset-1 columns">
<button class="btn grn-btn m-t-0" type="" ng-click="ok()">Ok</button>
</div>
<div class="col-sm-5 col-sm-offset-1 columns">
<button class="btn grn-btn line-btn m-t-0" type="" ng-click="cancelDelete()">Cancel</button>
</div>
</div>
</form>
</div>
</div>
</script>
</html>
Now you just need to inject $modal into your controller.
angular.module('app')
.controller('AdminListController', function($scope,$modal ) {
//code
});
Now add this code into your controller.
In this code I am simply calling the template through the id which I have used the modal template. And two functions are called for cancel and ok through which the modal will get closed as per the function.
$scope.Confirm = function ( ) {
alertModalInstance = $modal.open({
animation: $scope.animationsEnabled,
templateUrl: 'confirmAlert.html',
scope: $scope
});
$scope.cancelDelete = function () {
console.log("cancel");
alertModalInstance.dismiss('cancel');
};
$scope.ok = function () {
console.log("ok");
alertModalInstance.close(true);
};
0 Comment(s)