Theming allows you to change color of complete application from one place. In Angular Material , themes can be configured using $mdThemingProvider in application configuration.
Important terms used in Theming
1. Hues/ Shades
It is a single color within a palette
2. Palettes
Palette is a collection of hues/shades. Valid palettes include:
red , pink ,purple , deep-purple, indigo, blue, light-blue, cyan, teal, green, light-green, lime, yellow, amber, orange, deep-orange, brown, grey, blue-grey
3. Color Intentions
Color intention is a mapping of a palette .Valid color intention are:
primary, accent, warn
4. Themes
A theme is a configuration of palettes used for specific color intentions.
Classes used for theming:
md-button
md-checkbox
md-progress-circular
md-progress-linear
md-radio-button
md-slider
md-switch
md-tabs
md-input-container
md-toolbar
Configuring theme:
Theme is configured by using $mdThemingProvider. We can give color palette for each of the configuration method.
We can set a color for theme.primaryPalette, theme.accentPalette, theme.warnPalette, theme.backgroundPalette
as given below:
angular.module('myApp', ['ngMaterial'])
.config(function($mdThemingProvider) {
$mdThemingProvider.theme('default')
.primaryPalette('blue')
.accentPalette('light-blue')
.warnPalette('cyan')
.backgroundPalette('purple');
$mdThemingProvider.alwaysWatchTheme(true);
});
Disable theme:
We can disable theme by calling method disableTheming() as given below:
angular.module('myApp', ['ngMaterial'])
.config(function($mdThemingProvider) {
$mdThemingProvider.disableTheming();
});
Example of theme:
<link href="~/Content/angular-material.min.css" rel="stylesheet" />
<body ng-app="myApp">
<div ng-controller="themeController as crtl" md-theme="{{theme}}" md-theme-watch="true">
<md-input-container>
<label>Change theme</label>
<md-select ng-model="selectedTheme" ng-change="changeTheme()">
<md-option ng-value="theme" ng-repeat="theme in themes">{{ theme.name }}</md-option>
</md-select>
</md-input-container>
<div>
<md-button href="#" class="md-raised md-primary">
Primary
</md-button>
<md-button href="#" class="md-raised md-accent">
Accent
</md-button>
<md-button href="#" class="md-raised md-warn">
Warn
</md-button>
<md-button href="#" class="md-raised md-background">
Background
</md-button>
</div>
</div>
</body>
<script src="~/Scripts/angular.min.js"></script>
<script src="~/Scripts/angular-animate.min.js"></script>
<script src="~/Scripts/angular-aria.js"></script>
<script src="~/Scripts/angular-messages.min.js"></script>
<script src="~/Scripts/angular-material/angular-material.min.js"></script>
<script>
(function () {
var app = angular.module('myApp', ['ngMaterial'])
.controller('themeController', themeController)
.config(function ($mdThemingProvider) {
$mdThemingProvider.theme('themeRed')
.primaryPalette('red')
.accentPalette('pink')
.warnPalette('purple')
.backgroundPalette('orange');
$mdThemingProvider.theme('themeGreen')
.primaryPalette('green')
.accentPalette('light-green')
.warnPalette('lime')
.backgroundPalette('teal');
$mdThemingProvider.theme('themeBlue')
.primaryPalette('blue')
.accentPalette('light-blue')
.warnPalette('cyan')
.backgroundPalette('purple');
// to cascade down the changes through the DOM.
$mdThemingProvider.alwaysWatchTheme(true);
})
function themeController($scope) {
$scope.themes = [
{ name: 'Theme Red', value: 'themeRed' },
{ name: 'Theme Green', value: 'themeGreen' },
{ name: 'Theme Blue', value: 'themeBlue' },
];
$scope.selectedTheme = { name: 'Theme Red', value: 'themeRed' };
$scope.changeTheme = function () {
$scope.theme = $scope.selectedTheme.value;
};
}
})();
</script>
OUTPUT:
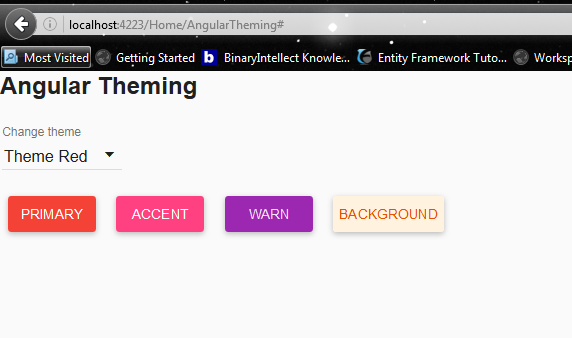
Hope this example will help you. Thanks
0 Comment(s)