Versioning ASP.NET Core Web API using HTTP Header Approach
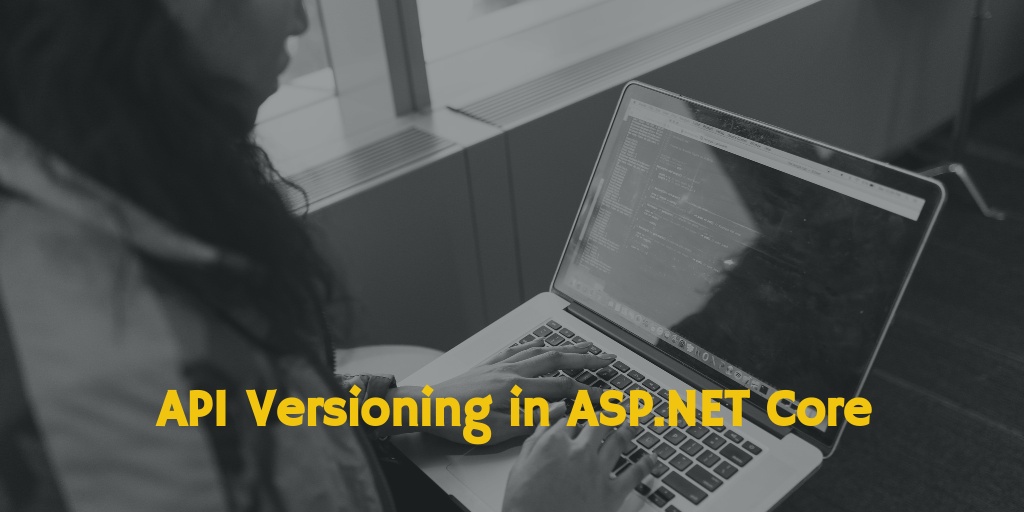
API versioning allows us to introduce new features in existing Web API and makes it more flexible and adaptable to the changes while keeping the existing functionalities intact. Imagine a situation where you have different customers who are using your Web API and you want to provide some additional features to those users who have upgraded their subscription. Besides, while implementing this change, you wouldn't like to change the URL. In such scenarios, Web API versioning would be an excellent solution.
Different ways of accessing Web API versions
Following are the ways to access all available versions of the Web API
-
Using query string
-
Using URL path segment
-
Using HTTP headers
In this article, we are going to have a look at the method of versioning ASP.NET Core Web API using HTTP header approach because it doesn't require changes in the URL to target different versions.
Step by step guide to creating a simple ASP.NET Core Web API with versioning
The code snippets that you will see in the following steps are created and tested using Visual Studio 2015 Community Edition and Microsoft .NET Core 1.0.1 VS 2015 Tooling Preview 2.
Step 1) Open Visual Studio and Create a new ASP.NET Core Web Application. Provide a suitable name and path for the application.
Step 2) Choose the template for your project. Select Web API and click OK.
Step 3) Now, add a versioning middleware Microsoft.AspNetCore.Mvc.Versioning in the application via a NuGet Package. Right-click on your project in Solution Explorer then select Manage NuGet Packages. In the NuGet Package Manager screen, switch to the Browse tab and search for Microsoft.AspNetCore.Mvc.Versioning. Now select it from the list and click on install button to install it.
Let's configure versioning middleware in our application with the help of ConfigureServices method in Startup.cs file.
public void ConfigureServices(IServiceCollection services)
{
// Add framework services.
services.AddApplicationInsightsTelemetry(Configuration);
// Setting configuration options for API versioning middleware
services.AddApiVersioning(options =>
{
options.ReportApiVersions = true;
options.AssumeDefaultVersionWhenUnspecified = true;
options.ApiVersionReader = new HeaderApiVersionReader("api-version");
options.DefaultApiVersion = new Microsoft.AspNetCore.Mvc.ApiVersion(2, 0);
});
services.AddMvc();
}
Let's take a look at the options we have used in the above code.
ReportApiVersions: When set to true, response header contains information about the supported versions.
AssumeDefaultVersionWhenUnspecified: This option allows a request to be served without specifying the version number. In our case, default API version will be 2.0.
ApiVersionReader: It is used to define the way client will pass the API version to the server. We have set it to HeaderApiVersionReader which allows the API version to be specified using HTTP header (api-version).
DefaultApiVersion: This property will define the default API version to be used when request header doesn't contain any version detail.
Step 4) We will add as many versions of Controller folders in our project. In this article, we are going to have two versions of API (1.0 and 2.0), added to two Controller folders (Controller1 and Controller2). Now, add a new Web API Controller Class file inside each Controller folder, keeping the default name as it is i.e, ValuesController.cs.
Step 5) Let's create simple Get API with different versions inside each Controller folder. In the following code snippets, API version is defined using [ApiVersion] attribute.
Get method with version 1.0 in ValuesController.cs file inside Controller1 folder
using Microsoft.AspNetCore.Mvc;
namespace ApiVersioningInCore.Controllers1
{
[ApiVersion("1.0")]
[Route("api/[controller]")]
public class ValuesController : Controller
{
[HttpGet]
public IActionResult Get() => Ok(new string[] { "value 1 from API version 1.0" });
}
}
Get method with version 2.0 in ValuesController.cs file inside Controller2 folder
using Microsoft.AspNetCore.Mvc;
namespace ApiVersioningInCore.Controllers2
{
[ApiVersion("2.0")]
[Route("api/[controller]")]
public class ValuesController : Controller
{
[HttpGet]
public IActionResult Get() => Ok(new string[] { "value 2 from API version 2.0" });
}
}
Testing versioning with different scenarios
Testing the APIs along with the version numbers is very important.
Run your Web API and open Postman tools to evaluate the results.
Test Scenario 1
When hitting API without specifying any header for version, we get output "value 2 from API version 2.0" because we have configured DefaultApiVersion to 2.0 in ConfigureServices method inside Startup.cs file.
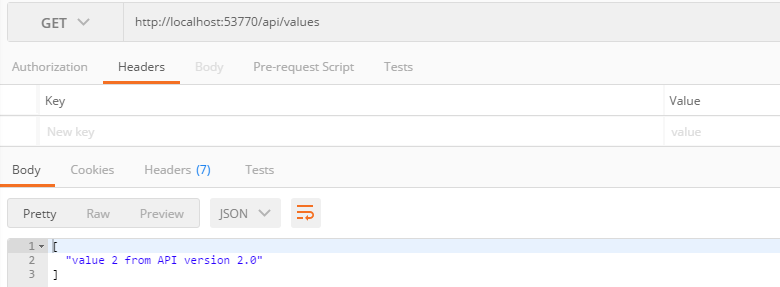
Test Scenario 2
Make another request with api-version header pointing to 1.0, and the output will be from 1.0 version i.e, "value 1 from API version 1.0"
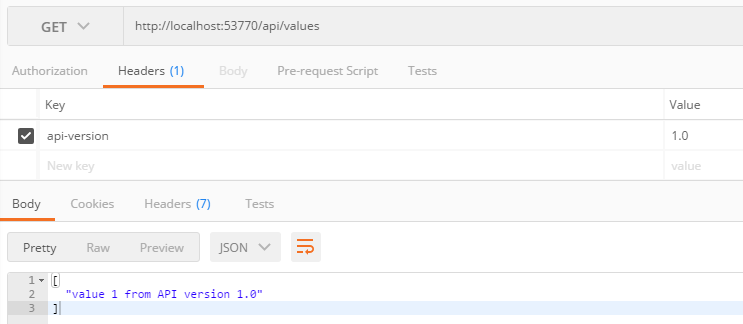
Test Scenario 3
Similarly, on making request with api-version header pointing to 2.0, we will get output from 2.0 version i.e, "value 2 from API version 2.0"
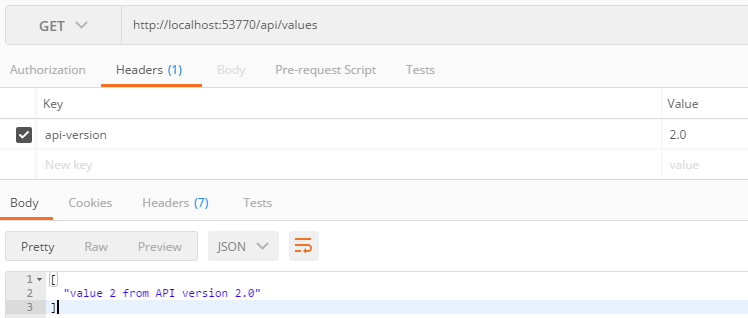
Test Scenario 4
You must be wondering what will happen if we specify a version in request header that doesn't really exist (for eg. 4.0). Well, in that case we will get the following exception.
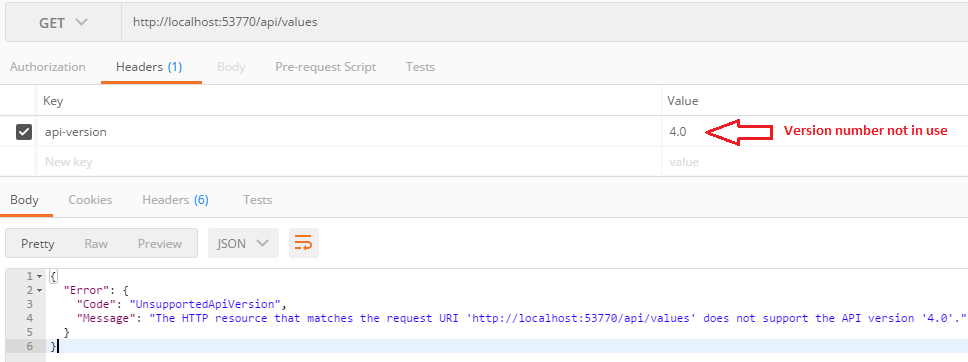
Checking supported API versions
The details about the supported API versions can be seen in the response header (api-supported-versions), given that ReportApiVersions is set to true in ConfigureServices method (Startup.cs file).
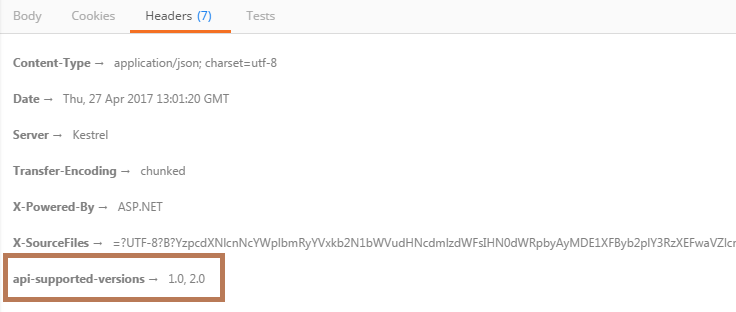
Hope it helps you. If you like the article, please share it on Facebook, LinkedIn, Twitter. Feel free to discuss anything about API versioning in the comment section below.
0 Comment(s)