Parse XML Documents by XMLDocument and XDocument
It is not a common practice to directly work on xml documents. In ASP.NET MVC whenever there is raw XML document available,to use them parsing is required. For parsing XML documents .Net provides two utility classes "XMLDocument" and "XDocument". Assembly reference System.Xml is to be used while using "XMLDocument" and assembly reference System.Xml.Linq is to be used when xml document is parsed using "XDocument". LINQ to XML uses XDocument for adding attributes,elements etc while XMLDocument does not provide LINQ to XML for same thing.
Below is the xml file named "StudentInfo.xml" in the location "D:/StudentInfo.xml".
<?xml version="1.0" encoding="utf-8" ?>
<StudentsInformation>
<GeneralInformation>
<UniversityName>XYZ University</UniversityName>
<Department>IT</Department>
</GeneralInformation>
<Studentlist>
<Student id="1" enrollment="4/30/2005">Suraj</Student>
<Student id="2" enrollment="3/4/2006">Depak</Student>
<Student id="3" enrollment="1/20/2011">Jyoti</Student>
<Student id="4" enrollment="1/20/2001" >Deepti</Student>
</Studentlist>
</StudentsInformation>
For parsing above xml document following steps is to be followed.
Step 1 : Create a mvc project in visual studio 2015.
Step 2 : In Models folder create following classes :-
namespace XMLParsingExample.Models
{
public class Student
{
public int id { get; set; }
public string name { get; set; }
public string enrollment { get; set; }
}
public class StudentInfo
{
public string University { get; set; }
public string Department { get; set; }
public List<Student> Studentlist { get; set; }
public StudentInfo()
{
University = "N/A";
Department = "N/A";
Studentlist = new List<Student>();
}
}
}
In above defined classes information is related to "StudentInfo.xml".
Step 3 : Create a controller named "Home" with action methods "ParsingByXmlDocument" and "ParseByXDocument".
using System;
using System.Web.Mvc;
using XMLParsingExample.Models;
using System.Xml;
namespace XMLParsingExample.Controllers
{
public class HomeController : Controller
{
private static string xmlUrl = @"D:/StudentInfo.xml";
// Parse the XML document using "XMLDocument"
public ActionResult ParsingByXmlDocument()
{
var student = new StudentInfo();
XmlDocument xmldoc = new XmlDocument();
//Load the XML file "xmlUrl" in the XMLDocument
xmldoc.Load(xmlUrl);
XmlNode StuInfoNode = xmldoc.SelectSingleNode("StudentsInformation/GeneralInformation");
student.University = StuInfoNode.SelectSingleNode("UniversityName").InnerText;
student.Department = StuInfoNode.SelectSingleNode("Department").InnerText;
XmlNode StuListNode = xmldoc.SelectSingleNode("StudentsInformation/Studentlist");
XmlNodeList StuNodeList = StuListNode.SelectNodes("Student");
foreach(XmlNode node in StuNodeList)
{
Student stu = new Student();
stu.id = Convert.ToInt16(node.Attributes.GetNamedItem("id").Value);
stu.enrollment = node.Attributes.GetNamedItem("enrollment").Value;
stu.name = node.InnerText;
student.Studentlist.Add(stu);
}
return View("Studentlist", student);
}
// Parse the XML document using "XDocument"
public ActionResult ParseByXDocument()
{
var student = new StudentInfo();
XDocument xdoc = XDocument.Load(xmlUrl); ;
XElement xelement = xdoc.Element("StudentsInformation").Element("GeneralInformation");
student.University = xelement.Element("UniversityName").Value;
student.Department = xelement.Element("Department").Value;
student.Studentlist = (from stu in xdoc.Descendants("Student")
select new Student()
{
id = Convert.ToInt16(stu.Attribute("id").Value),
name = stu.Value,
enrollment = stu.Attribute("enrollment").Value,
}).ToList<Student>();
return View("Studentlist", student);
}
}
}
View "Studentlist" is as follows where parsed xml is passed.
@model XMLParsingExample.Models.StudentInfo
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Studentlist</title>
</head>
<body>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.University)
</th>
<th>
@Html.DisplayNameFor(model => model.Department)
</th>
</tr>
<tr>
<td>
@Html.DisplayTextFor(model => model.University)
</td>
<td>
@Html.DisplayTextFor(model => model.Department)
</td>
</tr>
<tr>
<th>
@Html.DisplayName("StudentName")
</th>
<th>
@Html.DisplayName("StudentEnrollment")
</th>
</tr>
@foreach (var item1 in Model.Studentlist)
{
<tr>
<td>
@Html.DisplayTextFor(model => item1.name)
</td>
<td>
@Html.DisplayTextFor(model => item1.enrollment)
</td>
</tr>
}
</table>
</body>
</html>
Whenever the project runs with url "Home/ParsingByXmlDocument" or "Home/ParseByXDocument" the output remain same as they are just two methods of parsing xml document in asp.net mvc.
Output :
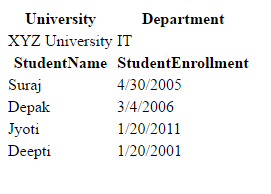
0 Comment(s)