Hello Readers !!
Today we are going to discuss the Facebook integration in the Unity Apps/Games.
Facebook integration is very common things in today apps. Now each and every app has Facebook section in it. So for the success of App now it's mandatory to app Facebook Invite and Facebook Share.
So today we are going to learn about this only.
Before to start work on that you need to have a Facebook app because you need to add Facebook id in the game.
So once you create a Facebook App then you can download the SDK from the following link:-
https://developers.facebook.com/docs/unity/downloads
You just need to add this sdk in the Unity.
Once you successfully added this SDK then you will see in your upper panel there is a Facebook Tag like below:-
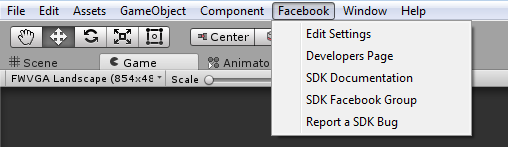
Just click on the "Edit Setting" then
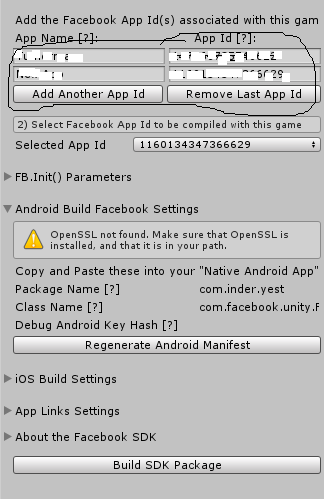
You need to add your id in the above circled section.
Then use following code to Login, Share and Invite.
using UnityEngine;
using System.Collections;
using Facebook.Unity;
using System.Collections.Generic;
public class FBManager : MonoBehaviour
{
string userName, emailID;
void Start ()
{
if (!PlayerPrefs.HasKey ("Yes"))
PlayerPrefs.SetString ("Yes", "NotLogin");
string _abc = PlayerPrefs.GetString ("Yes");
if (_abc == "Login")
{
FB.Init (Initcallback, OnHideUnity);
FB.ActivateApp ();
}
}
void OnGUI ()
{
if (!FB.IsLoggedIn) {
if (GUI.Button (new Rect (0, 0, 100, 100), "Login")) {
GetFBLogin ();
}
}
else
{
if (GUI.Button (new Rect (0, 0, 100, 100), "Log-Out")) {
FB.LogOut();
}
}
if (GUI.Button(new Rect(0, 150, 100, 100), "Share"))
{
FB.ShareLink(new System.Uri("https://play.google.com/apps"),
"Inder SIngh",
"Hello Inder Singh",
new System.Uri("https://plus.google.com/photos/photo/116421506444930084669/6327063379490630370?icm=false&authkey=CPHjtbzj_avxUw"),
callback: ShareCallback
);
}
if (GUI.Button(new Rect(0, 300, 100, 100), "Invite"))
{
FB.Mobile.AppInvite(new System.Uri("https://fb.me/1059246064189583"), callback: HandleInviteResult);
/*FB.AppRequest(
"Inder",
null,
null,
1,
"",
"",
"",
callback: onInviteComplete
);*/
//FB.AppRequest("INder",null,null,null,1,"","",onInviteComplete);
}
//
GUI.Label(new Rect(100,100,1000,1000), result);
}
private void onInviteComplete(IAppRequestResult result1)
{
result += "\n.... Invited Sent... ";
result += "\n"+result1.Cancelled.ToString();
}
string result = "Start";
private void HandleInviteResult (IAppInviteResult result1)
{
// result += "\nInvited Sent... ";
// result += "\n" + result1.Cancelled.ToString ();
if (result1.Cancelled.ToString () == "True")
{
Debug.Log("Request Send");
result += "\nInvited Sent... ";
}
else
result += "\nInvited Cancel... ";
//Debug.Log("Invited Cancel");
}
private void ShareCallback(IShareResult result1)
{
if (result1.Cancelled || !string.IsNullOrEmpty(result1.Error))
{
result += "\nShareLink Cancel... 111";
Debug.Log("ShareLink Error: " + result1.Error);
}
else if (!string.IsNullOrEmpty(result1.PostId))
{
result += "\nShareLink Cancel... 2222";
// Print post identifier of the shared content
Debug.Log(result1.PostId);
}
else
{
result += "\nShareLink Susses !... ";
// Share succeeded without postID
Debug.Log("ShareLink success!");
}
result += "\n" + result1.ToString ();
}
public void GetFBLogin()
{
if (!FB.IsInitialized)
{
//Initialize the fb SDK
FB.Init(Initcallback, OnHideUnity);
}
else
{
// Already initialized, signal an app activation App Events
FB.ActivateApp();
var parms = new List<string>() { "public_profile", "email" };
FB.LogInWithReadPermissions(parms, AuthCallBack);
}
}
// Reply from FB... after the initialization
private void Initcallback()
{
if (FB.IsInitialized)
{
// Debug.Log ("Initialized..........//");
// Signal an app activation App Events
FB.ActivateApp();
// Continue with Fb SDK..........
var parms = new List<string>() { "public_profile", "email" };
FB.LogInWithReadPermissions(parms, AuthCallBack);
}
else
{
Debug.Log("Failed to Initialize the Facebook SDK...");
}
}
// Controls the focus of the user on game..
private void OnHideUnity(bool isGameShown)
{
if (!isGameShown)
{
//Pause the game : we will need to hide
Debug.Log("No focus..........//");
Time.timeScale = 0;
}
else
{
// Resume the game : we're getting focus again
Debug.Log("focus..........//");
Time.timeScale = 1;
}
}
// logged-in the user and checks the generated tokens for user
private void AuthCallBack(ILoginResult result)
{
if (FB.IsLoggedIn)
{
PlayerPrefs.SetString ("Yes", "Login");
FB.API("/me?fields=first_name", HttpMethod.GET, DisplayUsername);
}
else
{
Debug.Log("user cancelled Login.....");
}
}
void DisplayUsername(IResult result1)
{
if (result1.Error == null)
{
result += "\n User Name :- "+result1.ResultDictionary["first_name"].ToString();
Debug.Log(result1.ResultDictionary["first_name"].ToString());
// FB.API("/me?fields=email", HttpMethod.GET, DisplayEmail);
}
else
{
Debug.Log(result1.Error);
}
}
void DisplayEmail(IResult result)
{
if (result.Error == null)
{
Debug.Log(result.ResultDictionary["email"].ToString());
}
}
}
Hope this blog will help you.
Try this and Keep Coding...
0 Comment(s)