In this tutorial, we will learn about "How to Fetch Facebook Photo Album Using PHP Application in Your Website". I have created a script where you can add your PAGE ID name to fetch all your Facebook albums in your website. With the help of this script, a new image will automatically fetch in your website whenever you post on your Facebook page. To achieve this, first you have to create Facebook app from Facebook developer page to get APP ID and APP Secret key.
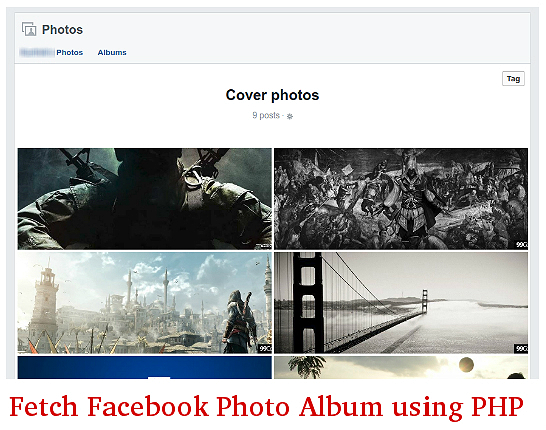
Now let's see the code part:
Java Script Code:
Enter the page id: <input type="text" id="m" value="">
<button type="button" id="myButton">submit</button>
<script>
var n = document.getElementById("m");
document.getElementById("myButton").addEventListener("click", function() {
document.location.href='facebook.php?fb='+n.value;
});
</script>
In the Below code, we’ll retrieve photo albums from the Facebook page and display in the webpage.
For that, you need to mention your newly created facebook APP ID ($app_id), APP Secret ($app_secret) in the below file (facebook.php).
Also, mention your facebook page id ($facebook_page_id).
PHP code:
<?php
if(!session_id()){
session_start();
}
if(isset($_SESSION['access_token'])){
// Get access token from session
$access_token = $_SESSION['access_token'];
}else{
// Facebook app id & app secret
$appId = 'xxxxxxxYour_APP_ID_xxxxxxxxxxxxx';
$appSecret = 'xxxxxxxYour_APP_Secret_xxxxxxxxxxxxx';
// Generate access token
$graphActLink = "https://graph.facebook.com/oauth/access_token?client_id={$appId}&client_secret={$appSecret}&grant_type=client_credentials";
// Retrieve access token
$accessTokenJson = file_get_contents($graphActLink);
$accessTokenObj = json_decode($accessTokenJson);
$access_token = $accessTokenObj->access_token;
// Store access token in session
$_SESSION['facebook_access_token'] = $access_token;
}
// Get photo albums of Facebook page using Facebook Graph API
$fields = "id,name,description,link,cover_photo,count";
$fb_page_id = $_GET['fb'];
$graphAlbLink = "https://graph.facebook.com/v2.9/{$fb_page_id}/albums?fields={$fields}&access_token={$access_token}";
$jsonData = file_get_contents($graphAlbLink);
$fbAlbumObj = json_decode($jsonData, true, 512, JSON_BIGINT_AS_STRING);
// Facebook albums content
$fbAlbumData = $fbAlbumObj['data'];
?>
In the Below code, we are using a foreach loop to fetch those Facebook albums which is active in your page.
(for example: Profile album, Timeline album & Cover album etc )
PHP code:
<?php
// Render all photo albums
echo "<br/><br/>";
foreach($fbAlbumData as $data){
$id = isset($data['id'])?$data['id']:'';
$name = isset($data['name'])?$data['name']:'';
$description = isset($data['description'])?$data['description']:'';
$link = isset($data['link'])?$data['link']:'';
$cover_photo_id = isset($data['cover_photo']['id'])?$data['cover_photo']['id']:'';
$count = isset($data['count'])?$data['count']:'';
$pictureLink = "photos.php?album_id={$id}&album_name={$name}";
echo "<a href='{$pictureLink}'>";
$cover_photo_id = (!empty($cover_photo_id ))?$cover_photo_id : 123456;
echo "<img width=100px height=100px src='https://graph.facebook.com/v2.9/{$cover_photo_id}/picture?access_token={$access_token}' alt=''>";
echo "</a>";
echo "<p>{$name}</p>";
$photoCount = ($count > 1)?$count. 'Photos':$count. 'Photo';
echo "<p><span style='color:#888;'>{$photoCount} / <a href='{$link}' target='_blank'>View on Facebook</a></span></p>";
echo "<p>{$description}</p>";
}
?>
In the Below code, we’ll retrieve all Photos from each album.
<link href="https://owlcarousel2.github.io/OwlCarousel2/assets/owlcarousel/assets/owl.carousel.min.css" rel="stylesheet"/>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://owlcarousel2.github.io/OwlCarousel2/assets/owlcarousel/owl.carousel.js"></script>
<?php
if(!session_id()){
session_start();
}
// Get album id from url
$album_id = isset($_GET['album_id'])?$_GET['album_id']:header("Location: facebook.php");
$album_name = isset($_GET['album_name'])?$_GET['album_name']:header("Location: facebook.php");
// Get access token from session
$access_token = $_SESSION['facebook_access_token'];
// Get photos of Facebook page album using Facebook Graph API
$graphPhoLink = "https://graph.facebook.com/v2.9/{$album_id}/photos?fields=source,images,name&access_token={$access_token}";
$jsonData = file_get_contents($graphPhoLink);
$fbPhotoObj = json_decode($jsonData, true, 512, JSON_BIGINT_AS_STRING);
// Facebook photos content
$fbPhotoData = $fbPhotoObj['data'];
?>
<?php echo "<h2>".$album_name."</h2>"; ?>
<div id="owl-demo" class="owl-carousel owl-theme">
<?php
// Render all photos
foreach($fbPhotoData as $data){
$imageData = end($data['images']);
$imgSource = isset($imageData['source'])?$imageData['source']:'';
$name = isset($data['name'])?$data['name']:'';
echo "<div class='item'>";
echo "<img src='{$imgSource}' alt=''>";
echo "<p>{$name}</p>";
echo "</div>";
}
?>
</div>
<script>
$(document).ready(function() {
$("#owl-demo").owlCarousel({
autoPlay: 3000,
items : 5,
itemsDesktop : [1199,3],
itemsDesktopSmall : [979,3]
});
});
</script>
<style>
#owl-demo .item{
margin: 3px;
}
#owl-demo .item img{
display: block;
width: 100%;
height: 350px;
}
</style>
Download code from here: https://github.com/pant24/facebook-album
Download code from command: git clone https://github.com/pant24/facebook-album
Demo:
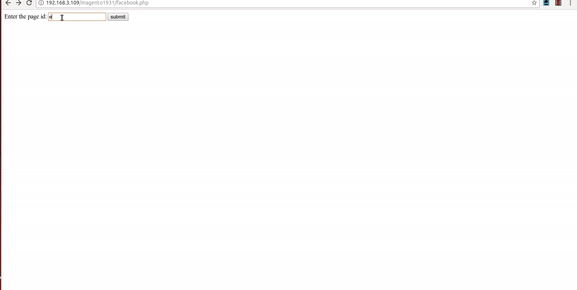
0 Comment(s)