Related pieces of functionality are grouped into angular module. The process of loading these functionality on demand is lazy loading. Lazy loading enables the application not to load everything at once instead allows requirement loading which application user wants when application first loads thus helps to decrease application startup time. Lazily loaded modules are loaded whenever user navigates to their routes.
Example to show lazy loading of angular module
Consider a root module "AppModule", parent component "AppComponent", and a component "HomeComponent".
app/app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
import { HomeComponent } from './home/home.component';
@NgModule({
declarations: [
AppComponent,
HomeComponent,
],
imports: [
BrowserModule,
AppRoutingModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
app/app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<div><button routerLink="home">Home</button><button routerLink="admin">Admin</button></div><router-outlet></router-outlet>'
})
export class AppComponent {
title = 'app';
}
app/app.component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
template: '<div><button routerLink="home">Home</button><button routerLink="admin">Admin</button></div><router-outlet></router-outlet>'
})
export class AppComponent {
title = 'app';
}
app/home/home.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-home',
template: '<p>Home Component within root module</p>',
})
export class HomeComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
Create a feature module using command "ng generate module admin --routing". It will create an admin module with a admin routing file. Create dashboard component within admin module using command "ng generate component dashboard".
app/admin/admin.module.ts
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { AdminRoutingModule } from './admin-routing.module';
import { DashboardComponent } from './dashboard/dashboard.component';
@NgModule({
imports: [
CommonModule,
AdminRoutingModule
],
declarations: [DashboardComponent]
})
export class AdminModule { }
app/admin/dashboard/dashboard.component.ts
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-dashboard',
template: '<p>Dashboard Component within admin module</p>',
})
export class DashboardComponent implements OnInit {
constructor() { }
ngOnInit() {
}
}
app/admin/admin-routing.module.ts
This is feature module navigation file where default is to display DashboardComponent.
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { DashboardComponent } from './dashboard/dashboard.component';
const routes: Routes = [
{ path:'',component : DashboardComponent }
];
@NgModule({
imports: [RouterModule.forChild(routes)],
exports: [RouterModule]
})
export class AdminRoutingModule { }
There is a root navigation file "app-routing.module.ts" which is the file used for configuration of routes.
app/app-routing.module.ts
import { NgModule } from '@angular/core';
import { Routes, RouterModule } from '@angular/router';
import { HomeComponent } from './home/home.component';
const routes: Routes = [
{
path: '',
redirectTo: 'home',
pathMatch: 'full'
},
{
path: 'admin',
loadChildren: 'app/admin/admin.module#AdminModule'
},
{
path: 'home',
component: HomeComponent,
},
];
@NgModule({
imports: [RouterModule.forRoot(routes)],
exports: [RouterModule]
})
export class AppRoutingModule { }
This file consist of two paths "home" and "admin". Default path is home which is using component property and navigates to "HomeComponent" while the other path admin causes lazy loading of admin module. To lazily load a module following are some key points :-
- loadChildren property is used in place of component.
- To avoid eagerly loading of module for loadChildren property string is passed instead of symbol.
- The string passed in loadChildren property not only defines path to module but also name of module class.
RouterModule.forRoot(routes) is in app-routing.module.ts where RouterModule let angular know that "AppRoutingModule" is a routing module and calling method forRoot makes it root routing module. It allows configuration of all routes passed to it, registers RouterService and makes router directives accessible. forRoot mehod can only be used once in application that too at root level. Whenever a routing module is created for feature module forChild method call is used no matter module is eagerly or lazily loaded. This lets angular know that route list through forChild is only providing additional routes and are useful for submodules and lazy loaded modules which need not to be loaded at application start. forChild method can be used in multiple modules but it does not include RouterService.
index.html
<!doctype html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>Angular5Demo</title>
<base href="/">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="icon" type="image/x-icon" href="favicon.ico">
</head>
<body>
<app-root></app-root>
</body>
</html>
When application runs following output is obtained
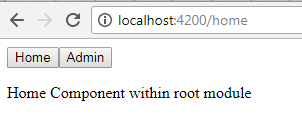
Explanation :- When application runs for first time the root module "AppModule" along with parent component "AppComponent" is loaded in browser and root navigation system causes default navigating component "HomeComponent" template to be displayed in place of <router-outlet></router-outlet> within AppComponent template.
Till this point, admin module is not downloaded, it is loaded only when button "admin" is clicked which downloads needed code. AdminModule navigation system causes default navigating component "DashboardComponent" template replacing <router-outlet></router-outlet> within AppComponent template and thus displaying DashboardComponent template data . Thus a module is lazily loaded.
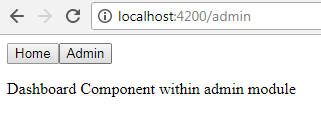
0 Comment(s)