Strictly Typed Arrays
Strictly Typed Arrays allows to define a variable number of arguments to a function by using "..."
We have to use "..." inside the method parentheses so that method accepts a variable length of arguments.
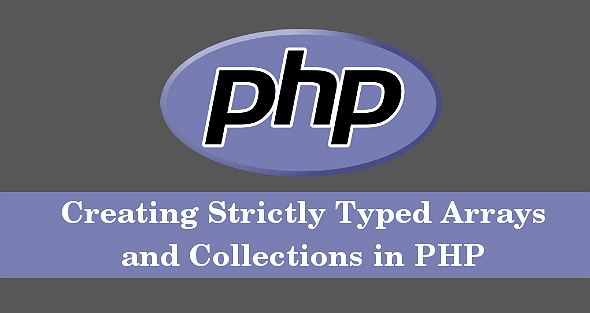
In the below example, we have passed variable number to the sum method which accept these variables with the help of "..." token.
<?php
function sum(...$numbers) {
$acc = 0;
foreach ($numbers as $n) {
$acc += $n;
}
return $acc;
}
echo sum(1, 2, 3, 4);
?>
Output: 10
Collection
Collection represent the correct OOP (Object Oriented programming) approach rather than using arrays. It is OO replacement for array data structure.
Collection also contains member elements similar to array but collection store any kind of data type such as strings and integers.
In other words collection is a class which is hold data of specific type or any type. Some collection may hold only string while other collections may hold strings, doubles and integer.
Creating A Collection.
$collection = new Collection(); //create the collection
$collection->add('ABC');
$collection->add('1 2 3');
Using add() function, we can add items to the collection, lets see how:
<php
$collection = new Collection(); //create the collection
$collection->addWithName('employee','pankaj');
$collection->addWithName('designation','software developer');
$collection->addWithName('year','Aug 2015');
?>
Using addWithName() function, we can add an item by its name.
In the above example, we had added a item with a key=>value relationship. Here we have set the keys that will be used to access the collection & value will be stored in the collection associated with the key.
Retrieve the value stored in the collection based on the key:
<?php
echo "<h1>Employee Information<h1>"
echo "<p>Employee name: ' .$collection.get('employee') .'</p>"
echo "<p>designation: ' .$collection.get('designation') .'</p>"
echo "<p>Joining Year: ' .$collection.get('year') .'</p>"
?>
output:
Employee Information
Employee name: pankaj
designation: software developer
Joining Year: Aug 2015
Hope you liked the tutorial, for any queries and input feel free to write in comment section below.
0 Comment(s)