NSPredicate is a query language. It provides a natural language interface to define logical conditions. While working with large data sets, sometimes it is essential to filter the data. So, the filtering and sorting of data can be done with NSPredicate. Let’s take an example to understand this :-
In the below example, we are going to display a contact list format i.e displaying names alphabetically with section title as the first letter. If a name starting with, lets say “Z” is not present then the section title will not be shown i.e “Z” title will not be there.
Lets start by dragging a UITableView in the Main.Storyboard and then a prototype cell on the table. After that give the identifier of the cell in the attributes inspector.
In your ViewController.m file include the following lines of code :-
@interface ViewController ()
{
NSArray *allNamesArray; // create an array of names
NSMutableArray *letters;
NSMutableDictionary *names;
}
@end
- (void)viewDidLoad {
[super viewDidLoad];
letters = [NSMutableArray new];
names = [NSMutableDictionary new];
allNamesArray = @[@"Anjali",@"Ankit",@"Jyoti",@"Sukanya",@"Tanuja",@"Smriti",@"Deepika"];
for (int i=65; i<=91; i++) {
NSString *charac=[NSString stringWithFormat:@"%c",i];
NSPredicate *predicate = [NSPredicate predicateWithFormat:@"SELF beginswith[c] %@",charac];
NSLog(@" First Letter %@",predicate);
NSArray *filteredArr= [allNamesArray filteredArrayUsingPredicate:predicate];
NSLog(@" Filtered array %@",filteredArr);
if (filteredArr.count>0) {
[letters addObject:charac];
[names setObject:filteredArr forKey:charac];
}
}
}
-(NSInteger)numberOfRows:(NSInteger )section
{
NSString *sectionTitle = [letters objectAtIndex:section];
NSArray *sectionContent = [names objectForKey:sectionTitle];
return [sectionContent count];
}
- (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView
{
// Return the number of sections.
return [names count];
}
- (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return [self numberOfRows:section];
}
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"cellIdentifier" forIndexPath:indexPath];
// Configure the cell...
NSString *sectionTitle = [letters objectAtIndex:indexPath.section];
NSArray *sectionContent = [names objectForKey:sectionTitle];
NSString *category = [sectionContent objectAtIndex:indexPath.row];
cell.textLabel.text = category;
cell.textLabel.textColor = [UIColor redColor];
return cell;
}
- (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section
{
if ([self numberOfRows:section] == 0) // If there is data in rows then only show the title header otherwise return nil
{
return nil;
} else {
return [letters objectAtIndex:section];
}
}
Build the program. You can see the console output as well as in the Simulator screen as :-
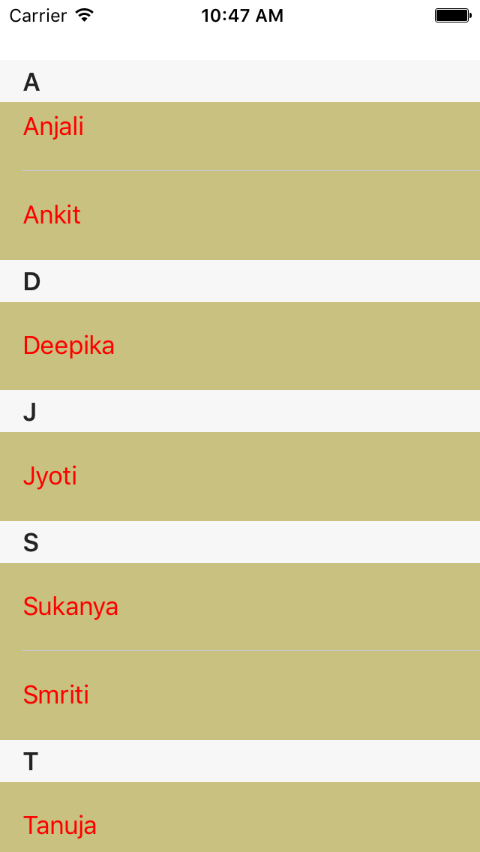
0 Comment(s)