An angular application is made up of components. A component view children can be defined as elements which are used within the component template(view). These elements can be directives, child components or name of template variable. @ViewChild and @ViewChildren decorator is used for referencing elements from the components view DOM. So, in this tutorial, I am providing you "An Overview of ViewChild & ViewChildren Decorator in Angular 4 with Example".
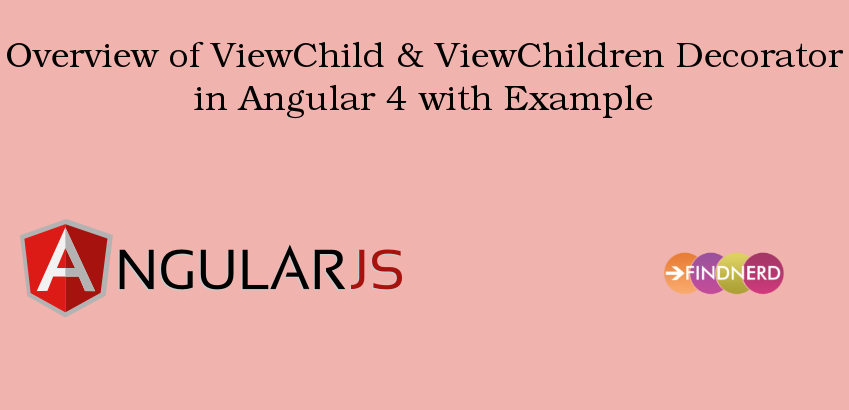
@ViewChild Decorator
Example: @ViewChild(StudentComponent) studentViewChild: StudentComponent;
It means search inside parent component template(view) for this child component and creates a property studentViewChild and after searching child component bind to this property. Thus property studentViewChild is storing the reference of child component(StudentComponent). If more than one StudentComponent are found inside parent component view then @ViewChild decorator only returns the first one it finds.
Example :-
Parent Component
import { Component,ViewChild,AfterViewInit } from '@angular/core';
import { StudentComponent } from './student/student.component';
@Component({
selector: 'app-root',
template: '<h1 #header>Parent Component</h1><app-student></app-student><app-student studenttype="Science Student"></app-student><app-student studenttype="Commerce Student"></app-student>'
})
export class AppComponent implements AfterViewInit {
@ViewChild("header") header
@ViewChild(StudentComponent) studentViewChild: StudentComponent
ngAfterViewInit() { //access view members only after the view is initially rendered
console.log(this.header);
console.log(this.studentViewChild);
}
}
Student Component
import { Component,Input } from '@angular/core';
@Component({
selector: 'app-student',
template: `<h1>{{studenttype}}</h1>`,
})
export class StudentComponent {
@Input() studenttype: string = "Humanities Student";
}
The following output is produced in console when application runs :-
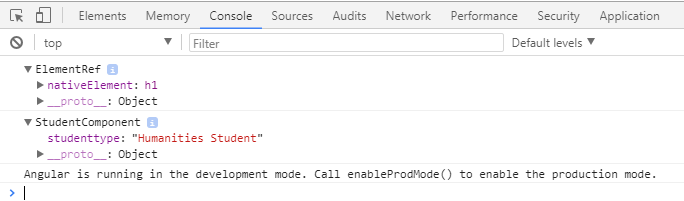
Explanation: When the parameter passed to @ViewChild is of type component/directive then the value returned is component/directive instance but in case of the name of a template variable as a parameter then the value returned is a reference to the native element. In StudentComponent @Input variable is set whose default value is "Humanities Student" otherwise the value will be one passed from the parent component. Only one reference to StudentComponent is returned because @ViewChild is only capable of returning the first element found against the searched element.
@ViewChildren Decorator
@ViewChild only returns the first element it finds corresponding to search element therefore not useful in case of multiple elements found corresponding to search element. @ViewChildren is used to get elements or directives Querylist from view DOM. Whenever parent component adds, removes or moves child element, Querylist will be automatically updated and will provide new value.
Example :-
Parent Component
import { Component,ViewChildren,AfterViewInit,QueryList } from '@angular/core';
import { StudentComponent } from './student/student.component';
@Component({
selector: 'app-root',
template: '<app-student></app-student><app-student studenttype="Science Student"></app-student><app-student studenttype="Commerce Student"></app-student>'
})
export class AppComponent implements AfterViewInit {
@ViewChildren(StudentComponent) studentViewChild: QueryList<StudentComponent>
ngAfterViewInit() {
let students: StudentComponent[] = this.studentViewChild.toArray();
console.log(students);
}
}
StudentComponent remains same
QueryList is the return type of @ViewChildren storing a list of items. The following output is produced in console when application runs:
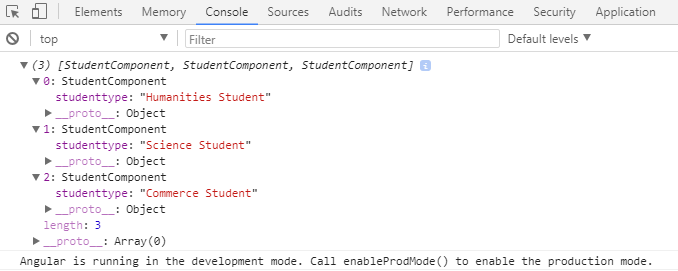
0 Comment(s)