Paging in ASP.NET MVC Application
To implement paging on an ASP.NET MVC Application following steps are to followed :-
Step 1 : Create a new C# ASP.NET Web project named Student_Management_System. Select the MVC template option in new ASP.NET Project dialog box and then click ok to create project.
Step 2 : PagedList.MVC should be installed from NuGet Package. This package helps in paging by providing various methods and properties. A paging helper named "PagedListPager" also gets installed by this package which helps in displaying paging buttons in views. A collection type named PagedList and various kind of extension methods are installed by PagedList package for IQueryable and IEnumerable collections.
In visual studio 2015 from Tools menu select NuGet package Manager and then Package Manager Console. In the Package Manager Console window run command "Install-Package PagedList.Mvc".
Step 3 :Create a Model named Student
using System.ComponentModel.DataAnnotations;
namespace Student_Management_System.Models
{
public class Student
{
[Required]
public string Fname { get; set; }
[Required]
public string Lname { get; set; }
[Required]
public string Email { get; set; }
public Student(string fname, string lname, string email)
{
this.Fname = fname;
this.Lname = lname;
this.Email = email;
}
}
}
Step 4 : Create a controller with an action method Details containing paging functionality.
using System;
using System.Collections.Generic;
using System.Web.Mvc;
using Student_Management_System.Models;
using PagedList; //for usage of PagedList package
namespace Student_Management_System.Controllers
{
public class HomeController : Controller
{
public ActionResult Details(int? page)
{
int pageSize = 3;
int pageNumber = (page ?? 1);
List<Student> students = new List<Student>();
students.Add(new Student("suraj","rana","s@gmail.com"));
students.Add(new Student("jyoti","pandey","j@gmail.com"));
students.Add(new Student("deepak","rana", "d@gmail.com"));
students.Add(new Student("anita","rawat", "s@gmail.com"));
students.Add(new Student("aditi","rawat", "a@gmail.com"));
students.Add(new Student("anupam","rawat", "anu@gmail.com"));
students.Add(new Student("arvind","singh", "arv@gmail.com"));
students.Add(new Student("megha", "nawani", "megha@gmail.com"));
return View(students.ToPagedList(pageNumber,pageSize));
}
}
}
In the above action method an argument page is passed which defines current page number. If the value of passed argument page is null then "??" null-coalescing will return a default value 1 else value of page variable is returned to pageNumber variable.
Step 5 : Create a view corresponding to action method Details containing paging links.
@model PagedList.IPagedList<Employee_Management_System.Models.Student>
@using PagedList.Mvc;
<link href="~/Content/PagedList.css" rel="stylesheet" type="text/css" />
@{
Layout = null;
}
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>Details</title>
</head>
<body>
<table class="table">
<tr>
<th>
@Html.DisplayName("First Name")
</th>
<th>
@Html.DisplayName("Last Name")
</th>
<th>
@Html.DisplayName("Email")
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Fname)
</td>
<td>
@Html.DisplayFor(modelItem => item.Lname)
</td>
<td>
@Html.DisplayFor(modelItem => item.Email)
</td>
</tr>
}
</table>
Page@(Model.PageCount < Model.PageNumber ? 0 : Model.PageNumber) of @Model.PageCount
@Html.PagedListPager(Model, page => Url.Action("Details", new { page }))
</body>
</html>
The @model statement signifies that view will get PagedList object.
The PagedList.Mvc with using statement signifies an access to the MVC helper( PagedListPager ) which displays paging buttons.
Output :
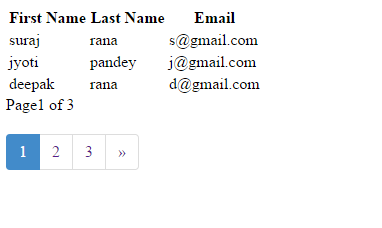
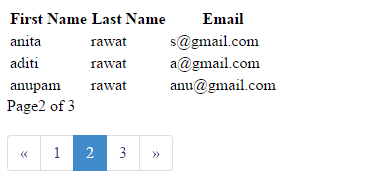
1 Comment(s)