There are different ways for passing data between controllers in Objective-C. In this example we are going to use NSNotificationCenter for passing data from one view controller to another view controller. NSNotificationCenter is generally used to broadcast or send message within a program. We post a notification at one place and when the task is done it is shown in the desired place.
Let’s take an example to understand this. In this example we are going to take two ContainerView in the ViewController. In one ContainerView we will take a SegmentControl and on clicking different segments the background color of other ContainerView will change.
To start with, drag two ContainerView in the ViewController in Main.storyboard. You can see two new ViewControllers are also included with the ContainerView. In one of the container drag a SegmentControl. Create two classes and assign them to both ContainerView. Create the IBAction of the SegmentControl.
Include the following lines of code to the action of the UISegmentControl from where we are posting the notification :-
- (IBAction)btnSegmentControl:(UISegmentedControl *)sender {
NSString * noticationName = @"notificationName";
switch (sender.selectedSegmentIndex) {
case 0:{
UIColor *brownColor = [UIColor brownColor];
[[NSNotificationCenter defaultCenter] postNotificationName:noticationName object:brownColor];
break;
}
case 1:{
UIColor *grayColor = [UIColor grayColor];
[[NSNotificationCenter defaultCenter] postNotificationName:noticationName object:grayColor];
break;
}
}
}
Now to receive notification in the next ContainerView include the following lines of code :-
- (void)viewDidLoad {
[super viewDidLoad];
NSString *notificationName = @"notificationName"; // the notification name must be the same as in the postNotification method
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(useNotificationWithString:)name:notificationName object:nil];
}
- (void)useNotificationWithString:(NSNotification *)notification // selector method definition
{
[self.view setBackgroundColor:[notification object]];
}
- (void)dealloc {
[[NSNotificationCenter defaultCenter] removeObserver:self];
}
Below is the output of the code :-
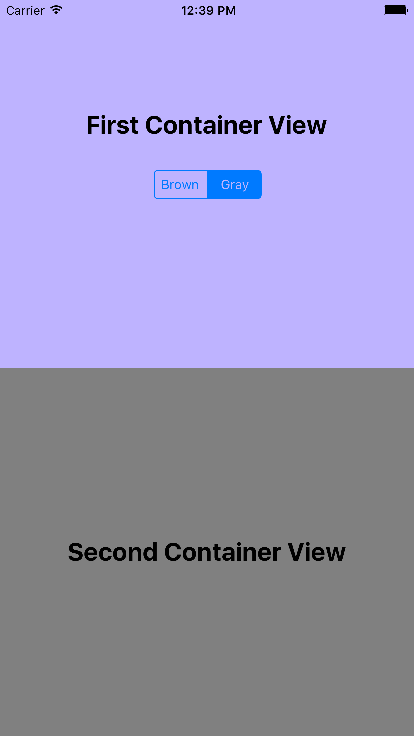
0 Comment(s)