The Room is basically an easier implementation of SQLite database and a part of Android Architecture components.
We know that SQLite uses SQLite open helper class to manage SQLite database but Room provides migration by removing this implementation.
In SQLite database, we basically use content values to map data into a table or construct queries and then uses the cursor to get data one by one but in case if we add incorrect column name in the query then runtime exception occurs and it takes more time to identify the syntax error.
But Room library provides you an easy interface to implement a database, by using Room you can check all the queries at runtime like for syntax error or column name error.
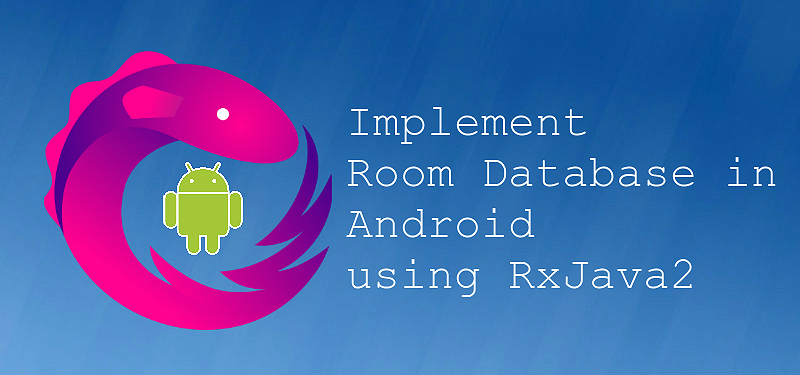
Now this example will show you how to use RxJava with Room library to provide an asynchronous mechanism.
Please follow the step by step procedure to implement Room with RxJava:
Step 1: Add dependencies in app.gradle :
compile "android.arch.lifecycle:runtime:1.0.0-alpha5"
compile "android.arch.lifecycle:extensions:1.0.0-alpha5"
annotationProcessor "android.arch.lifecycle:compiler:1.0.0-alpha5"
compile "android.arch.persistence.room:runtime:1.0.0-alpha5"
annotationProcessor "android.arch.persistence.room:compiler:1.0.0-alpha5"
compile "android.arch.persistence.room:rxjava2:1.0.0-alpha5"
add maven in project gradle file :
allprojects {
repositories {
jcenter()
maven { url 'https://maven.google.com' }
}
}
Step 2: Declare a model class using Entity, ColumnInfo, Primary key annotation :
@Entity (tableName = "Students")
public class StudentModel {
//Declare student id as a primary key in database will auto generate by Room
@PrimaryKey (autoGenerate = true)
public int mId;
//Declare Column name in table Students
@ColumnInfo (name = "StudentName")
public String mName;
@ColumnInfo (name = "StudentClass")
public String mStd;
public int getmId() {
return mId;
}
public void setmId(int mId) {
this.mId = mId;
}
public String getmName() {
return mName;
}
public void setmName(String mName) {
this.mName = mName;
}
public String getmStd() {
return mStd;
}
public void setmStd(String mStd) {
this.mStd = mStd;
}
}
Step 3: Now create a Data access object class to define the queries using query, insert, delete annotation and it will return flowable, maybe, single type of data as an observable:
@Dao
public interface StudentDao {
//Flowable or observable is used to emits Student model types of data and it emits whenever database is updated
@Query("SELECT * FROM Students")
Flowable <List<StudentModel>> getStudents();
//This Maybe class is used to emit only a single row data
@Query("SELECT * FROM Students WHERE StudentName =:aStudentName ")
Maybe<StudentModel> getStudentByName(String aStudentName);
//Insert the parameters in table like list of students
@Insert
void insertStudent(ArrayList<StudentModel> mStudentModelArrayList);
//This Query will delete all the students from table Students
@Query("DELETE FROM Students")
void deleteAllStudents();
}
Step 4: Create abstract class that will extend Room database and will return Dao like this :
//Declare entities of your room database with version
@Database(entities = StudentModel.class,version = 1)
public abstract class StudentDatabase extends RoomDatabase{
public abstract StudentDao getStudentDao();
}
Step 5: Now insert and retrieve the data from Room database :
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Access Room database in activity to get data access object
StudentDatabase mStudentDatabase = StudentDatabase.getAppDatabase(MainActivity.this);
ArrayList<StudentModel> modelArrayList = new ArrayList<>();
//Saving some records
for (int i=0;i<10;i++){
StudentModel mStudentModel = new StudentModel();
mStudentModel.setmName("Name :"+i);
mStudentModel.setmStd("class :"+i);
modelArrayList.add(mStudentModel);
}
//inserting records
mStudentDatabase.getStudentDao().insertStudent(modelArrayList);
//getting flowable to subscribe consumer that will access the data from Room database.
mStudentDatabase.getStudentDao().getStudents().subscribe(new Consumer<List<StudentModel>>() {
@Override
public void accept(@NonNull List<StudentModel> studentModels) throws Exception {
handleResponse(studentModels);
}
});
}
private void handleResponse(List<StudentModel> studentModels){
Log.e("student size :",studentModels.size()+"");
for (int i=0;i<studentModels.size();i++){
Log.e("student name :",studentModels.get(i).getmName());
}
}
}
Hope you enjoy the tutorial ! If you have any question, feel free to write in comment section.
0 Comment(s)