In android, we simply hide a view by setting its visibility. We can do this either in programming or in xml.
In programming (we can say in Activity, Fragment etc) there is setVisibility(int) method to set or control the visibility of the view.
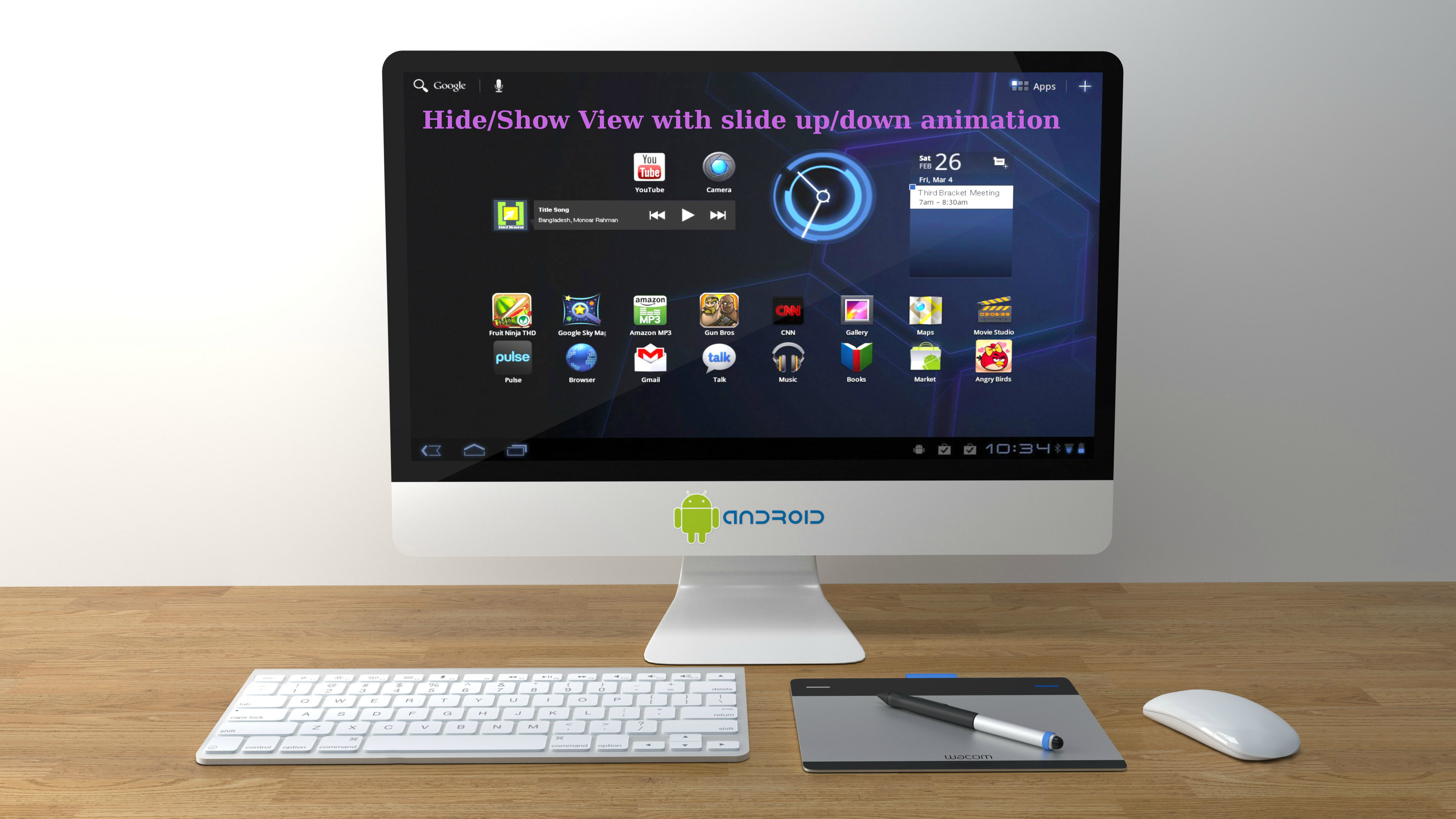
In this tutorial, we will see, How to Hide/Show a View with slide up/down animation in Android.
Example:-
To make view visible:-
view.setVisibility(View.VISIBLE)
To hide the view:-
view.setVisibility(View.GONE)
or
view.setVisibility(View.INVISIBLE)
Note:- GONE:- hides the view, and it doesn't take any space for layout purposes.
INVISIBLE:- hides the view, but it still takes up space for layout purposes.
To set visibility via xml we simply set the attribute android:visibility=""
Example:-
To make view visible:-
android:visibility="visible"
To hide the view:-
android:visibility="invisible"
or
android:visibility="gone"
Now we apply animation to set the visibility of a view.
1) Add view_hide.xml and view_show.xml in anim folder.
view_hide.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<translate
android:duration="1000"
android:fromYDelta="0"
android:toYDelta="100%p" />
</set>
view_show.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android" >
<translate
android:duration="1000"
android:fromYDelta="100%p"
android:toYDelta="0" />
</set>
Now in the Activity class, declare two reference of Animation class
private Animation animShow, animHide;
Initialize the references (animShow and animHide)
private void initAnimation()
{
animShow = AnimationUtils.loadAnimation( this, R.anim.view_show);
animHide = AnimationUtils.loadAnimation( this, R.anim.view_hide);
}
To show the view with slide up animation
view.setVisibility(View.VISIBLE);
view.startAnimation( animShow );
To hide the view with slide down animation
view.startAnimation( animHide );
view.setVisibility(View.GONE);
1 Comment(s)