TDD (Test-Driven-Development) is getting popular day by day defining its needs in greater code testing. This process is a test which we define to get an output by applying to our library, API etc. Day by day Nodejs is now developing as a language of choice for REST API development, and Express has become as a framework of choice.
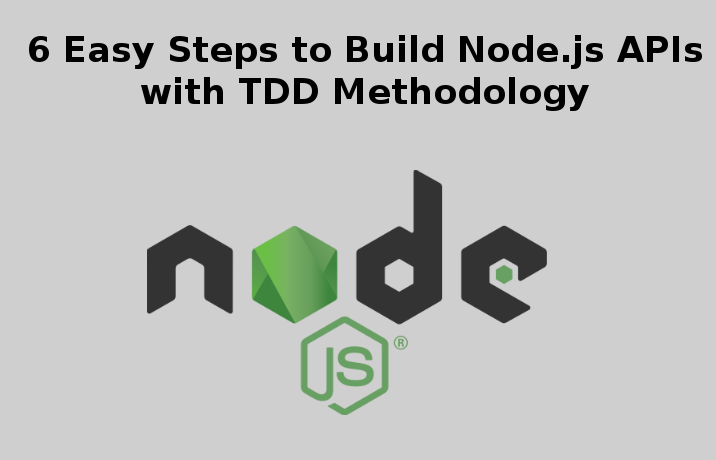
The first step is to test your APIs you create before building an Express project. lets see how:
STEP 1: Here, we are starting with a simple Express application that provide API functionality for addition and subtraction
- Run following in a terminal to download example application-
git clone https://github.com/cianclarke/tdd-for-apis.git ; cd tdd-for-apis # Clone the finished repository
git checkout boilerplate # jump to the initial project code
- Now, download dependencies that will be required further development process. Run following commands to set up test framework.
npm install --save-dev supertest
npm install --save-dev mocha
Once above step is done, we will have our testing framework ready. Now the next process to create our first integration test.
STEP 2: Creating Integration test
- Create a directory in which you will take test a nd blank file to store test file. For that first, run following commands form root directory
mkdir -p test/integration
touch test/integration/test-route-addition.js
- let ‘s, we will write an integration test, for that we have mount our route at /add and it will take 2 query string params called a and b. Our Expected output status should be 200(OK), if any values get missed or is non-integer value, then we receive status code 422 un-processable entity.
Our test will look like:
var supertest = require('supertest'),
app = require('../../app');
exports.addition_should_accept_numbers = function(done){
supertest(app)
.get('/add?a=1&b=1')
.expect(200)
.end(done);
};
exports.addition_should_reject_strings = function(done){
supertest(app)
.get('/add?a=string&b=2')
.expect(422)
.end(done);
};
- Run following command to run the subtraction test case;
touch test/integration/test-route-subtraction.js
var supertest = require('supertest'),
assert = require('assert'),
app = require('../../app');
exports.addition_should_respond_with_a_numeric_result = function(done){
supertest(app)
.get('/subtract?a=5&b=4')
.expect(200)
.end(function(err, response){
assert.ok(!err);
assert.ok(typeof response.body.result === 'number');
return done();
});
};
STEP 3: Run the integration tests
- In the downloaded code, You can find the files /add and /subtract which are already mounted in app.js, their defining routes can be found in /routes directory. The 200(OK) status code will be returned by these routes, this provides starting functionality-creating failing test, then implement.
Run following command:
node_modules/.bin/mocha -u exports test/integration/*
You can see all the changes for this step, including what npm does when we run `install --save-dev` on GitHub.
STEP 4: Make integration tests pass
- Now Let’s implement two routes, /addition and /subtraction, this will take number and return a the result in numeric form.
- Create lib directory with two files add.js and subtract.js, both of them will export function that will return 0 .Run the following commands:
mkdir lib
echo "module.exports = function(){ return 0; };" > lib/add.js
echo "module.exports = function(){ return 0; };" > lib/subtract.js
- Set up route handlers to use add and subtract function. In routes/add.js :
var add = require('../lib/add');
module.exports = function(req, res, next){
return res.json({ result : add(req.a, req.b) });
};
app.use(function(req, res, next){
var a = parseInt(req.query.a),
b = parseInt(req.query.b);
if (!a || !b || isNaN(a) || isNaN(b)){
return res.status(422).end("You must specify two numbers as query params, A and B");
}
req.a = a;
req.b = b;
return next();
});
STEP 5: Add unit tests
- Add unit tests to check if our addition and subtraction logic fails. Let ‘s create blank files for our addition and subtraction. Run following commands to create test files:
mkdir test/unit
touch test/unit/test-lib-addition.js ; touch test/unit/test-lib-subtraction.js;
- Addition test will look like this:
var assert = require('assert'),
add = require('../../lib/add');
exports.it_should_add_two_numbers = function(done){
var result = add(1,1);
assert.ok(result === 2);
return done();
};
exports.it_should_add_two_negative_numbers = function(done){
var result = add(-2,-2);
assert.ok(result === -4);
return done();
};
STEP 6: How to run tests by users:
- Add an entry to our package.json file like,
"scripts" : {
"test" : "node_modules/.bin/mocha -u exports test/**/*"
}
- Run our test by following command and it will show result :
npm test
1 Comment(s)