Today I am going to write a short note on setImmediate, hope you will understand how setImmediate works in nodejs.
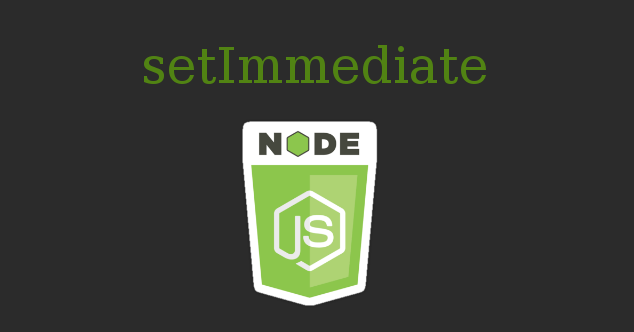
setImmediate
Once the current poll phase is completed, setImmediate will execute. After all the tasks has completed its execution in its event loop, then setImmediate will tell the browser to execute your code. SetImmediate can be cleared by clearImmediate(). By calling clearImmediate(), it will cancel the object created by setImmediate().
On browsers, setImmediate allow developers to break from long running tasks. As soon as browser completes its pending task from its event loop then it will receive a callback. This will increase the CPU utilization and consumes less power than the usual setTimeout() pattern.
Let us understand setImmediate by the following program:
setImmediate(function First() {
setImmediate(function Second() {
console.log("1");
setImmediate(function Fourth() { console.log(2); });
setImmediate(function Fifth() { console.log(3); });
});
setImmediate(function Third() {
console.log(4);
setImmediate(function Sixth() { console.log(5); });
setImmediate(function Seventh() { console.log(6); });
});
});
setTimeout(function timeout() {
console.log('TIMEOUT FIRED');
}, 0)
Following is the output of the above code
// 'TIMEOUT FIRED' 1 4 2 3 5 6
setImmediate callbacks are fired off the event loop, once per iteration in the order that they were queued.
Callback "First" is fired off from the first iteration of the event loop. Callback "Second" is fired on the second iteration of the event loop, Callback "Third()" is fired on the third iteration of the event loop. This prevents event loop from being blocked and allows other I/O or timer callbacks to be called in the mean time.
Non blocking code can be written using setImmediate(). Example: Write a program to sum the numbers from 1 to n. If the user input the number "4", then sum will be 1+2+3+4 = 10.
I have created small program using restify. You can install it using
npm install restify
Create server.js file and write the following program:
var restify = require('restify');
var server = restify.createServer();
function sumofnumber(req,res,next){
var number = req.params.number;
var sum = 0;
var counter = 1;
var recursion = function(counter,number){
setImmediate(function(){
if(counter<=number){
sum+=parseInt(counter);
counter++;
recursion(counter,number);
}else{
res.send("Sum = "+sum);
console.log(sum);
}
});
}
recursion(1,number);
}
server.listen(8080, function() {
console.log('%s listening at %s', server.name, server.url);
});
0 Comment(s)