Exception Handling in Rails using begin rescue
Exception is a condition, that occurs when something goes wrong in a code. Normally in that case the program gets terminated. In rails, an object containing the information of the error is an instance of Exception class, or one of class Exception's children. A sample hierarchy for Exception class is as given below in the diagram:
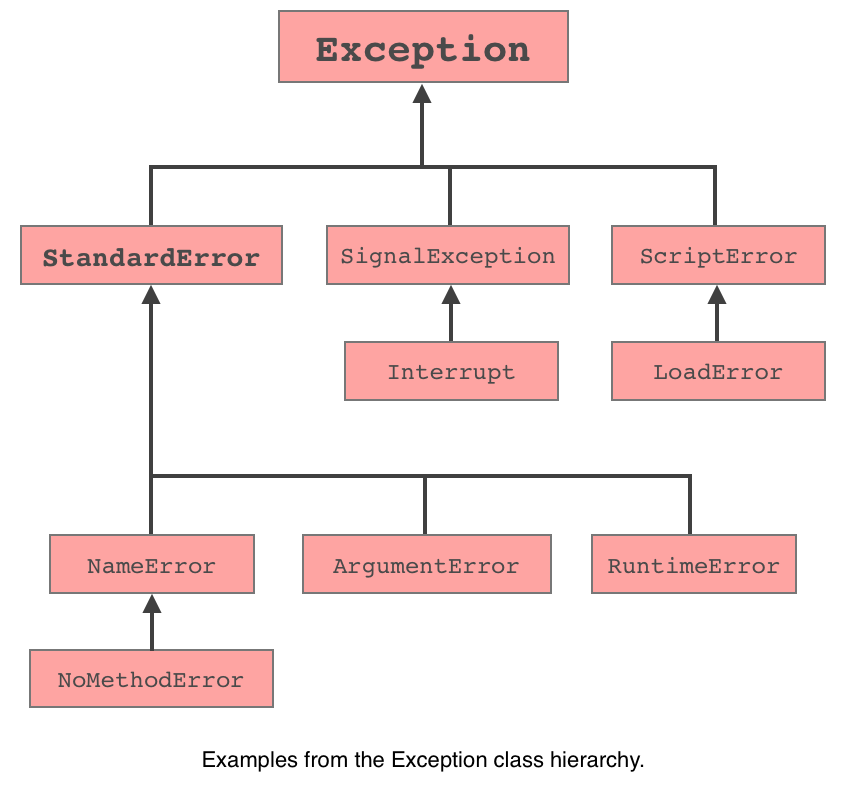
Now as we don't want the program to halt in any case, so we need to handle the exception. Rails provide an easy and effective way of handling exceptions. One of the way is using begin..rescue..end:
begin..rescue..end
In this approach whatever code we want to write we write it inside begin and rescue and we handle it inside rescue and end. beside rescue we can give the class of the exception or its superclass, then the rescue will handle only that kind of exception. A common syntax can be:
begin
# some code
rescue OneTypeException
# some code
rescue OtherTypeException
# some
else
# some other exceptions
ensure
# It will always get executed
end
Lets understand the above syntax, any code written between begin and rescue is monitored, so if the exception relates to the class specified with rescue class or its subclass then the thrown exception is handled. If there is no exception then the else block gets executed. The code inside ensure block always gets executed.
Example:
begin
file = open("no_file_exist")
rescue StandardError => e
print "Inside Exception Class\n"
print e + "\n"
print "\n"
else
print "Inside else block\n"
ensure
print "Inside ensure block\n"
end
#=> Output will be
Inside Exception Class
No such file or directory @ rb_sysopen - no_file_exist
Inside ensure block
Thus the call in the above example, first came to StandardError rescue block and also went to ensure block. Lets see if there is no exception then what will happen
begin
x = 10 + 5
print "Output is : " + x.to_s + "\n"
rescue StandardError => e
print "Inside Excetion Class\n"
print e
print "\n"
else
print "Inside else block\n"
ensure
print "Inside ensure block\n"
end
# Output will be:
Output is : 15
Inside else block
Inside ensure block
Thus we can see that here the whole code inside the begin-rescue block got executed but the rescue part got ommitted and again the else part and the ensure part got executed.
Hope you liked reading this blog.
0 Comment(s)