Databinding is an architecture that consist 4 major parts first is: source object that supplies the information to viewed pages, Second is: data consumer that displays the information, third is: agent that ensures that data is synchronised between the both and the fourth and last one is the table repetition agent, it works with data agent in tabular form like HTML tables elements.
So in this tutorial, I will explain the simple steps for implementing databinding in android, the code will let you to work with data-Binding process in Android.
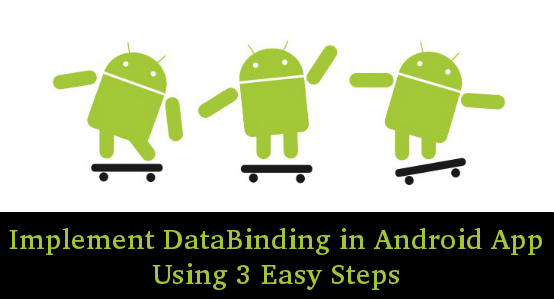
Step 1. First you need to add the below code snippet to your buid.gradle file to configure data binding in your android app
android {
....
dataBinding {
enabled = true
}
}
NOTE: You should be using Android Studio 1.3 or later version as these provide support for data-Binding
Step 2. Below is the code for my SignUpForm where user inserts the data FistName, LastName, UserName and Password .
private void init()
{
btnSubmit=(Button)findViewById(R.id.btnSubmit);
btnMove=(Button)findViewById(R.id.btnMove);
etFirstName=(EditText)findViewById(R.id.etFirstName);
etLastName=(EditText)findViewById(R.id.etLastName);
etUserNa=(EditText)findViewById(R.id.etUserName);
etPaswd=(EditText)findViewById(R.id.etSPassword);
etConferm=(EditText)findViewById(R.id.etSConferm);
btnSubmit.setOnClickListener(this);
btnMove.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
Intent intent=new Intent(SingnUpForm.this,DashBoard.class);
startActivity(intent);
}
});
}
@Override
public void onClick(View view) {
user=etUserNa.getText().toString();
first=etFirstName.getText().toString();
last=etLastName.getText().toString();
pwd=etConferm.getText().toString();
UserDetailBean userDetailBean=new UserDetailBean();
userDetailBean.setFirstName(etFirstName.getText().toString());
userDetailBean.setLastName(etLastName.getText().toString());
userDetailBean.setUserName(etUserNa.getText().toString());
userDetailBean.setPassword(etConferm.getText().toString());
etFirstName.setText("");
etLastName.setText("");
etUserNa.setText("");
etConferm.setText("");
etPaswd.setText("");
Toast.makeText(this, "Data Entered Successfully", Toast.LENGTH_SHORT).show();
Gson gson=new Gson();
String userDetail=gson.toJson(userDetailBean);
Utility.setPrefrences(this,"VALUE",userDetail);
}
Its xml file that is being inflated to this activity is below:
- activity_sign_up_form.xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:orientation="vertical">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/tvSignUp"
android:textSize="@dimen/fontSize"
android:textStyle="bold|italic"
android:layout_gravity="center_horizontal"/>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:weightSum="2">
<EditText
android:id="@+id/etFirstName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/etFirstName"
android:layout_weight="1"
/>
<EditText
android:id="@+id/etLastName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/etLastName"
android:layout_weight="1"
/>
</LinearLayout>
<EditText
android:layout_marginTop="@dimen/fontSize"
android:id="@+id/etUserName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="@string/etSUser"
/>
<EditText
android:layout_marginTop="@dimen/fontSize"
android:id="@+id/etSPassword"
android:layout_width="match_parent"
android:password="true"
android:layout_height="wrap_content"
android:hint="@string/etSPassword"
/>
<EditText
android:layout_marginTop="@dimen/fontSize"
android:id="@+id/etSConferm"
android:layout_width="match_parent"
android:password="true"
android:layout_height="wrap_content"
android:hint="@string/etSConferm"
/>
<Button
android:id="@+id/btnSubmit"
android:layout_marginTop="@dimen/fontSize"
android:layout_gravity="center_horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/btnSubmit"/>
<Button
android:textAllCaps="false"
android:id="@+id/btnMove"
android:layout_marginTop="@dimen/fontSize"
android:layout_gravity="center_horizontal"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Next Screen"/>
</LinearLayout>
I am saving all inserted data in string in Utility.java file making use of a UserDetailBean class.
public class Utility {
static String PREFERENCE_NAME="demo";
public static void setPrefrences(Context context, String tag, String value) {
SharedPreferences preferences = context.getSharedPreferences(PREFERENCE_NAME, MODE_PRIVATE);
SharedPreferences.Editor editor = preferences.edit();
editor.putString(tag, value);
editor.commit();
}
public static String getPrefrences(Context context, String tag) {
// Read
SharedPreferences preferences = context.getSharedPreferences(PREFERENCE_NAME, MODE_PRIVATE);
return preferences.getString(tag, "");
}
}
public class UserDetailBean {
private String FirstName;
private String LastName;
private String UserName;
private String Password;
public String getFirstName() {
return FirstName;
}
public void setFirstName(String firstName) {
FirstName = firstName;
}
public String getLastName() {
return LastName;
}
public void setLastName(String lastName) {
LastName = lastName;
}
public String getUserName() {
return UserName;
}
public void setUserName(String userName) {
UserName = userName;
}
public String getPassword() {
return Password;
}
public void setPassword(String password) {
Password = password;
}
}
Step 3. Now in DashBoard activity I'm showing all data with the help of DataBinding in simple words I'm using the databinding concept in this file. The xml layout for the databinding is little bit different as this xml file start with a root tag of layout followed by the data element. The variable within data displays the properties that are used within the layout mentioned below. The Expressions in the layout are written in attribute properties using "@{}
" syntax. See the activity_dash_board.xml below:
<?xml version="1.0" encoding="utf-8"?>
<layout xmlns:android="http://schemas.android.com/apk/res/android">
<data>
<variable name="user" type="com.databinding.android.databindingdemo.UserDetailBean"/>
</data>
<ScrollView
android:id="@+id/scrlv"
android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:orientation="vertical">
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:weightSum="2">
<TextView
android:text="FirstName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
/>
<TextView
android:text="LastName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
/>
</LinearLayout>
<LinearLayout
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:weightSum="2">
<TextView
android:text="@{user.FirstName}"
android:id="@+id/tvFirstName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
/>
<TextView
android:text="@{user.LastName}"
android:id="@+id/tvLastName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_weight="1"
/>
</LinearLayout>
<TextView
android:layout_marginTop="@dimen/fontSize"
android:text="UserName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<TextView
android:text="@{user.UserName}"
android:layout_marginTop="@dimen/fontSize"
android:id="@+id/tvUserName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<TextView
android:layout_marginTop="@dimen/fontSize"
android:text="Password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
<TextView
android:text="@{user.Password}"
android:layout_marginTop="@dimen/fontSize"
android:id="@+id/tvPassword"
android:layout_width="match_parent"
android:layout_height="wrap_content"
/>
</LinearLayout>
</ScrollView>
</layout>
- And below is the code for the DashBoard Activity that inflates the above layout
DashBoard.java
public class DashBoard extends AppCompatActivity {
private TextView tvFirstName, tvLastName, tvUserName, tvPassword;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
ActivityDashBoardBinding activityDashBoardBinding= DataBindingUtil.setContentView(this,R.layout.activity_dash_board);
Gson gson=new Gson();
UserDetailBean userDetailBean=gson.fromJson(Utility.getPrefrences(this,"VALUE"),UserDetailBean.class);
activityDashBoardBinding.setUser(userDetailBean);
}
}
In the above activity I have made use of the DataBinding class that is generated based on the name of layout file, here the file generated is named ActivityDashBoardBinding.
0 Comment(s)