Synchronous means to process something serially but in the Asynchronous way you can process data simultaneously you don't need to wait for the first process to be completed.
In Android app we basically hit API to fetch lots of data from the server then populates it in our app but in that time progress bar comes up on the screen and we can't interact with other parts of the app because it's just a synchronous mechanism. To provide an Asynchronous mechanism in our app we can use Reactive programming that is most popular nowadays.
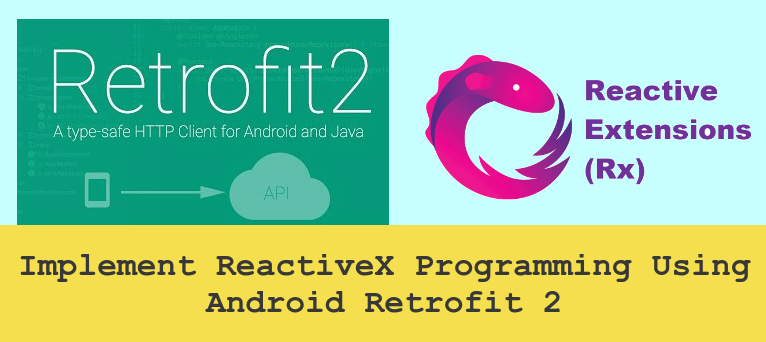
RxJava basically works on the Observable pattern to provide Asynchronous functionality.
There are two basic terms in RxJava that are Observable and Observer.
Observable - Observable is an object who emits the data asynchronously.
Observer - Observer is basically a consumer that consume or use the data emitted by observer.
But now the question is how can we provide asynchronous mechanism because working on the same thread or main thread can lead exceptions, for this scheduler is used to identify the observable thread and observer thread, in this way observable and observer both run on different threads.
Basically, we used scheduleOn and observeOn method to provide observable and observer thread.
Now its time to see the example on how to use Retrofit with RxJava for Reactive programming.
Step 1: First of all add the following dependencies :
compile 'com.squareup.retrofit2:retrofit:2.1.0'
compile 'com.squareup.retrofit2:converter-gson:2.1.0'
compile 'io.reactivex.rxjava2:rxjava:2.0.1'
compile 'io.reactivex.rxjava2:rxandroid:2.0.1'
compile 'com.jakewharton.retrofit:retrofit2-rxjava2-adapter:1.0.0'
we are using Retrofit2 and RxJava2 that is latest version.
Step 2: Enable jack option inside defaultConfig tag in your app gradle to use java8's Lambda expression
jackOptions {
enabled true
}
now add compile option inside android tag
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
Now build your project to use RxJava and other defined classes.
Step 3: Declare an interface for Retrofit Api like this:
public interface ApiInterface {
@GET("Your api end point")
Observable<List<StudentModel>> register();
//Here we are creating a method register that will return a Observable class to emit Student type data
}
Step 4: Create Model class
public class StudentModel {
private String id;
private String name;
public String getVer() {
return id;
}
public String getName() {
return name;
}
}
Step 5: Create RecyclerView and row Items like this:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.learn2crack.retrofitrxjava.MainActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/recycler_view"
android:scrollbars="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</RelativeLayout>
<android.support.v7.widget.CardView
xmlns:card_view="http://schemas.android.com/apk/res-auto"
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="5dp"
android:layout_marginLeft="5dp"
android:layout_marginRight="5dp"
card_view:cardCornerRadius="5dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="10dp"
android:orientation="vertical">
<TextView
android:id="@+id/tv_id"
android:layout_marginTop="10dp"
android:layout_marginBottom="10dp"
android:textSize="18sp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textStyle="bold" />
<TextView
android:id="@+id/tv_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
</android.support.v7.widget.CardView>
Step 6: Create Adapter class for recyclerView
public class DataAdapter extends RecyclerView.Adapter<DataAdapter.ViewHolder> {
private ArrayList<StudentModel> mStudentList;
public DataAdapter(ArrayList<StudentModel> studentList) {
mStudentList = studentList;
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.recycler_row, parent, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(ViewHolder holder, int position) {
holder.mTvName.setText(mStudentList.get(position).getName());
holder.mTvId.setText(mStudentList.get(position).getID());
}
@Override
public int getItemCount() {
return mStudentList.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
private TextView mTvName,mTvId;
public ViewHolder(View view) {
super(view);
mTvName = (TextView)view.findViewById(R.id.tv_name);
mTvId = (TextView)view.findViewById(R.id.tv_id);
}
}
}
Step 7:
public class MainActivity extends AppCompatActivity {
public static final String BASE_URL = "Your base url /";
//Declare base url
private RecyclerView mRecyclerView;
private CompositeDisposable mCompositeDisposable;
private DataAdapter mAdapter;
private ArrayList<StudentModel> mStudentArrayList;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mCompositeDisposable = new CompositeDisposable();
// Declare Composite Disposable i.e used to add multiple disposable and provide O(1) comlplexity for add and remove opearations.
mRecyclerView = (RecyclerView) findViewById(R.id.recycler_view);
mRecyclerView.setHasFixedSize(true);
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(getApplicationContext());
mRecyclerView.setLayoutManager(layoutManager);
fetchAndPopulateData();
}
private void fetchAndPopulateData() {
ApiInterface requestInterface = new Retrofit.Builder()
.baseUrl(BASE_URL)
.addCallAdapterFactory(RxJava2CallAdapterFactory.create())
// addCallAdapterFactory this is used to define the call adapter for supporting service method retun type
// RxJava2CallAdapterFactory this factory class basically creates observable, adding this here in Retrofit returns
// a observable declared in service class.
.addConverterFactory(GsonConverterFactory.create())
// just to add Gson convertor
.build()
.create(ApiInterface.class);
mCompositeDisposable.add(requestInterface.register()
// Adding Observable here by calling register method of api interface.
.observeOn(AndroidSchedulers.mainThread())
// Declaring a observer thread where data ll populate.
.subscribeOn(Schedulers.io())
// Declaring a Observable thread from where data will emit.
.subscribe(this::handleResponse)
// Subscribing or registering observervable source.
// Using Java 8 double comma operator to make it consumer implementation short otherwise it will be like this
/*
.subscribe(new Consumer<List<StudentModel>>() {
@Override
public void accept(List<StudentModel> studentModels) throws Exception {
handleResponse(studentModels);
}
})
*/
);
}
private void handleResponse(List<StudentModel> androidList) {
mStudentArrayList = new ArrayList<>(androidList);
mAdapter = new DataAdapter(mStudentArrayList);
mRecyclerView.setAdapter(mAdapter);
}
@Override
public void onDestroy() {
super.onDestroy();
mCompositeDisposable.clear();
// clear all disposable that was attached to activity.
}
}
Run and check the response and populate time it ll take less time than other mechanisms and will allow you to work on multiple thread asynchronously.
0 Comment(s)