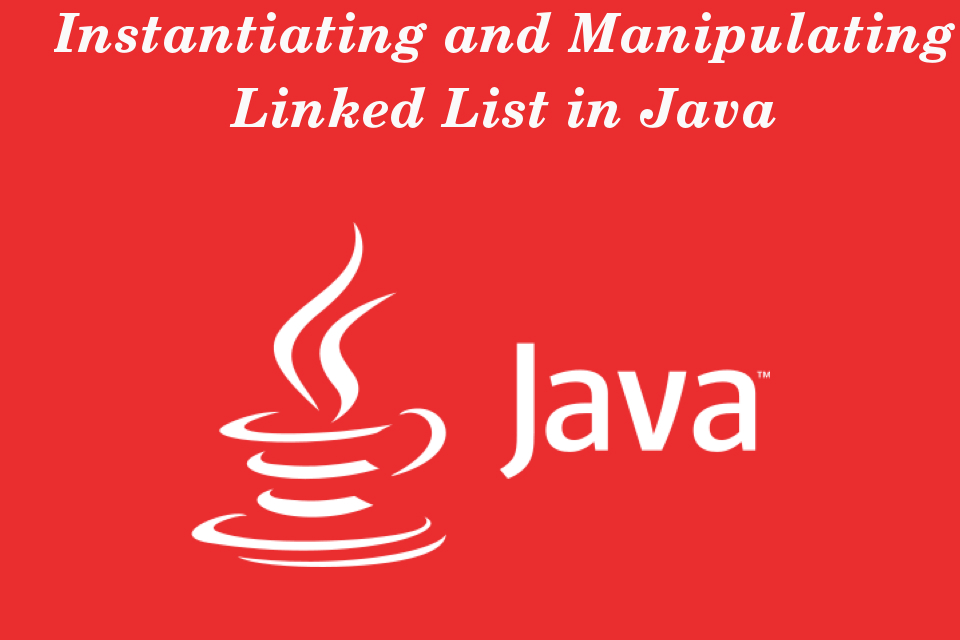
Introduction:
In this blog we will learn about LinkedList.In general terms, LinkedList is a data structure where each element consist of three parts. First part represents the link to the previous element, second part represents the value of the element and last one represents the next element. In java LinkedList is a collection class that can be used both as a Queue as well as a List. It can contain elements of any type either null or duplicate. We can remove and delete elements from either end and get any element from any position. Element in the LinkedList is known as node. LinkedList class extends AbstractSequentialList class and implements the interfaces Cloneable and Serializable.
Properties:
1- Insertion and removal is faster in LinkedList compared to ArrayList as we do not shift elements after insertion or removal we only change the references.
2- The process of retrieval of an element is time taking in LinkedList comared to ArrayList as there is a traversal process from beginning/end to end/beginning depending on which is closer to the element.
3- It has methods pop() and push() to work like stack.
4-It can be used as Single Linked List , Doubly Linked List, Queue or ArrayList.
5- It can consist of multiple null elements and duplicate elements.
6- Random Access is not allowed.
Methods used by LinkedList to manipulate the nodes of LinkedList:
Insertion at the start of LinkedList:
Examples using methods: add(E e), addFirst(E e), offerFirst(E e)
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// linked list creation
LinkedList demoll = new LinkedList();
// adding elements
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.addFirst("Kotdwar");
demoll.offerFirst("Uttarkashi");
Iterator irt = demoll.iterator();
int count = 1;
while(irt.hasNext()){
Object element = irt.next();
System.out.println("Element No "+ count +": " + element);
count++;
}
}
}
Output:
Element No 1: Uttarkashi
Element No 2: Kotdwar
Element No 3: Dehradun
Element No 4: Haridwar
Element No 5: Mussoorie
Element No 6: Rishikesh
Element No 7: Nainital
Insertion in the middle of LinkedList:
Examples using methods: add(int index, E e), addAll(int Index,collection c)
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// linked list object creation
LinkedList demoll = new LinkedList();
// adding elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath"); // adding elements to index 1 of the linked list
List newlist = new ArrayList();
newlist.add("listitem_1");
newlist.add("listitem_2");
newlist.add("listitem_3");
demoll.addAll(2,newlist);
Iterator itr = demoll.iterator();
int count = 1;
while(itr.hasNext()){
Object element = itr.next();
System.out.println("Element No "+ count +": " + element);
count++;
}
}
}
Output:
Element No 1: Dehradun
Element No 2: Joshimath
Element No 3: listitem_1
Element No 4: listitem_2
Element No 5: listitem_3
Element No 6: Haridwar
Element No 7: Mussoorie
Element No 8: Rishikesh
Element No 9: Nainital
Insertion at the end of LinkedList:
Examples using methods: add(E e), addAll(Collection c),offer(E e),offerLast(E e)
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
//linked list object creation
LinkedList demoll = new LinkedList();
// adding elements to the linked list at end of the existing last element
demoll.add("Dehradun");
demoll.add("Haridwar");
// adding elements to the linked list to the index 1
demoll.add(1,"Nainital");
demoll.add(1,"Joshimath");
// creating list object
List newlist = new ArrayList();
newlist.add("listitem_1");
newlist.add("listitem_2");
newlist.add("listitem_3");
demoll.addAll(newlist);
demoll.offer("Last_item_1"); //This will add an item to the end of Linkedlist
demoll.offerLast("Last_item_2"); //This will add an item to the end of Linkedlist
Iterator itr = demoll.iterator();
int count = 1;
while(itr.hasNext()){
Object element = itr.next();
System.out.println("Element No "+ count +": " + element);
count++;
}
}
}
Output:
Element No 1: Dehradun
Element No 2: Nainital
Element No 3: Haridwar
Element No 4: listitem_1
Element No 5: listitem_2
Element No 6: listitem_3
Element No 7: Last_item_1
Element No 8: Last_item_2
Removal of elements:
Removal of nodes from the head:
Examples using methods: Poll(), PoolFirst(), remove(), removeFirst()
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// creating linked list object
LinkedList demoll = new LinkedList();
// add elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath");
// creating list object
List newlist = new ArrayList();
newlist.add("listitem_1");
newlist.add("listitem_2");
newlist.add("listitem_3");
demoll.addAll(2,newlist);
demoll.poll(); // This will remove Dehradun from the list
demoll.pollFirst(); // This will remove Joshimath from the list
demoll.remove(); // This will remove listitem_1 from the list
demoll.removeFirst(); // This will remove listitem_2 from the list
Iterator itr = demoll.iterator();
int count = 1;
while(itr.hasNext()){
Object element = itr.next();
System.out.println("Element No "+ count +": " + element);
count++;
}
}
}
Output:
Element No 1: listitem_3
Element No 2: Haridwar
Element No 3: Mussoorie
Element No 4: Rishikesh
Element No 5: Nainital
Removal of nodes from the middle:
Examples using method: Remove(int index)
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// create a linked list object
LinkedList demoll = new LinkedList();
// add elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath");
// creating list object
List newlist = new ArrayList();
newlist.add("listitem_1");
newlist.add("listitem_2");
newlist.add("listitem_3");
demoll.addAll(2,newlist);
demoll.remove(4); //This will remove listitem_3 from the list
Iterator itr = demoll.iterator();
int count = 1;
while(itr.hasNext()){
Object element = itr.next();
System.out.println("Element No "+ count +": " + element);
count++;
}
}
}
Output:
Element No 1: Dehradun
Element No 2: Joshimath
Element No 3: listitem_1
Element No 4: listitem_2
Element No 5: Haridwar
Element No 6: Mussoorie
Element No 7: Rishikesh
Element No 8: Nainital
Removal nodes from the tail:
Examples using methods: PollLast(), RemoveLast().
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// create a linked list object
LinkedList demoll = new LinkedList();
// add elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath");
// List object creation
List newlist = new ArrayList();
newlist.add("listitem_1");
newlist.add("listitem_2");
newlist.add("listitem_3");
demoll.addAll(2,newlist); // This will add the all the list elements to the LinkedList at
index 2nd onwards
demoll.pollLast(); //This will remove Nainital from the list
demoll.removeLast(); //This will remove Rishikesh from the list
Iterator itr = demoll.iterator();
int count = 1;
while(itr.hasNext()){
Object element = itr.next();
System.out.println("Element No "+ count +": " + element);
count++;
}
}
}
Output:
Element No 1: Dehradun
Element No 2: Joshimath
Element No 3: listitem_1
Element No 4: listitem_2
Element No 5: listitem_3
Element No 6: Haridwar
Element No 7: Mussoorie
Retrieval of elements from the head:
Examples using methods:element(), getFirst(), peek(), PeekFirst()
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// creating a linked list object
LinkedList demoll = new LinkedList();
// adding elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath");
List newlist = new ArrayList();
newlist.add("listitem_1");
newlist.add("listitem_2");
newlist.add("listitem_3");
demoll.addAll(2,newlist);
System.out.println("The first element retrieved is :" + demoll.element());
System.out.println("The first element retrieved is :" + demoll.getFirst());
System.out.println("The first element retrieved is :" + demoll.peek());
System.out.println("The first element retrieved is :" + demoll.peekFirst());
}
}
Output for all the methods will be :
The first element retrieved is :Dehradun
The first element retrieved is :Dehradun
The first element retrieved is :Dehradun
The first element retrieved is :Dehradun
Retrieval of elements from the middle:
Example using method: get(int index)
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// creating a linked list object
LinkedList demoll = new LinkedList();
// add elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath");
System.out.println("The element retrieved in 3rd position is = " + demoll.get(3));
}
}
Output:
The element retrieved in 3rd position is = Mussoorie
Retrieval of nodes from the tail:
Example using methods: getLast(),peekLast()
import java.util.*;
public class DemoLinkedList {
public static void main(String args[]) {
// creating a linked list object
LinkedList demoll = new LinkedList();
// add elements to the linked list
demoll.add("Dehradun");
demoll.add("Haridwar");
demoll.add("Mussoorie");
demoll.add("Rishikesh");
demoll.add("Nainital");
demoll.add(1,"Joshimath");
System.out.println("The last node retrieved from the list= :" + demoll.getLast());
System.out.println("The last node retrieved from the list= :" + demoll.peekLast());
}
}
Output:
The last node retrieved from the list= Nainital
The last node retrieved from the list= Nainital
There are some more methods that we can use to manipulate Linkedlist.
1) void clear(): This method clears all the elements in a list.
demoll.clear();
2) Object clone(): It will return type is object hence it returns the copy of the list.
3) boolean contains(Object objitem): It returns true if the “objitem” is present in the list else false.
4) int indexOf(Object objitem): This method is used to get the index of the specific item in the list.
5) int lastIndexOf(Object objitem): This method is used to get the index of the last occurrence of the specific element.
6) Object set(int index, Object objitem): This method is used to update the value of the specified “index” with the give value “objitem”.
7) int size(): This method returns the total number of elements in the list.
0 Comment(s)