Dear programmers,
Please follow the following steps to create a simple Spring MVC project in your favourite IDE.
1. Create a dynamic web application (maven-archetype-webapp) project.
2. Add Spring dependencies :
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.evontech.blog</groupId>
<artifactId>spring-mvn</artifactId>
<packaging>war</packaging>
<version>0.0.1-SNAPSHOT</version>
<name>spring-mvn-resourse Maven Webapp</name>
<url>http://maven.apache.org</url>
<properties>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<springframework.version>4.1.6.RELEASE</springframework.version>
<jstl.version>1.2</jstl.version>
</properties>
<dependencies>
<!-- Spring -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>${springframework.version}</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<finalName>spring-mvn</finalName>
</build>
</project>
Please notice I've just added a single dependency for artifact spring-webmvc in dependencies. Maven download itself all its dependencies automatically so no need to define other explicitly.
3. Configure Spring Dispatcher Servlet in web.xml :
Dispatcher Servlet is the only method to send requests or receive responses through it to the web application. Dispatcher Servlet gets the requests with models, finds appropriate controller and responds in form of appropriate view.
<!DOCTYPE web-app PUBLIC
"-//Sun Microsystems, Inc.//DTD Web Application 2.3//EN"
"http://java.sun.com/dtd/web-app_2_3.dtd" >
<web-app>
<display-name>Sample Web Application</display-name>
<servlet>
<servlet-name>mvc</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>mvc</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
</web-app>
Please notice, Spring Dispatcher Servlet tries to load application context by finding it with the same name postfixed with '-servlet'. In the example above, it finds mvc-servlet.xml and loads the web application context from it.
4. Configure Application Context :
In the mvc-servlet.xml, we define the beans that helps Dispatcher Servlet in finding the right controller and the view.
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:context="http://www.springframework.org/schema/context"
xmlns:mvc="http://www.springframework.org/schema/mvc"
xsi:schemaLocation="http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.2.xsd
http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd">
<mvc:annotation-driven />
<mvc:resources location="/resources/" mapping="/resources/**" />
<context:component-scan base-package="com.evontech.blog" />
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix">
<value>/WEB-INF/jsp/</value>
</property>
<property name="suffix">
<value>.jsp</value>
</property>
</bean>
</beans>
In the XML above, I've enabled the Spring annotation feature. This feature allow Spring to find the annotated classes and work according to the annotations.
Spring comes with its own controller to handle the static resources like images, css and JS. And that is what <mvc:resource> does.
Let the spring know the package where to search annotated class using component-scan.
View Resolver helps to find the view against a request.
5. Controllers :
I've the controller like the following -
package com.evontech.blog;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class AppController {
@RequestMapping("/")
public String home(Model model) {
//business logic here
model.addAttribute("greeting", "Hello world !!");
return "home";
}
}
@controller annotation does two things -
1) Tells spring that its a type of controller. I'll react on requests.
2) Allows Spring to create an object inside application context.
As in the example above, AppController is just controlling request coming from '/'. And it returning the actual name of the view to load on this request.
6. View :
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8" isELIgnored="false"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Home</title>
</head>
<body>
${greeting}
</body>
</html>
7. Test :
Run the project with your favourite servlet container.
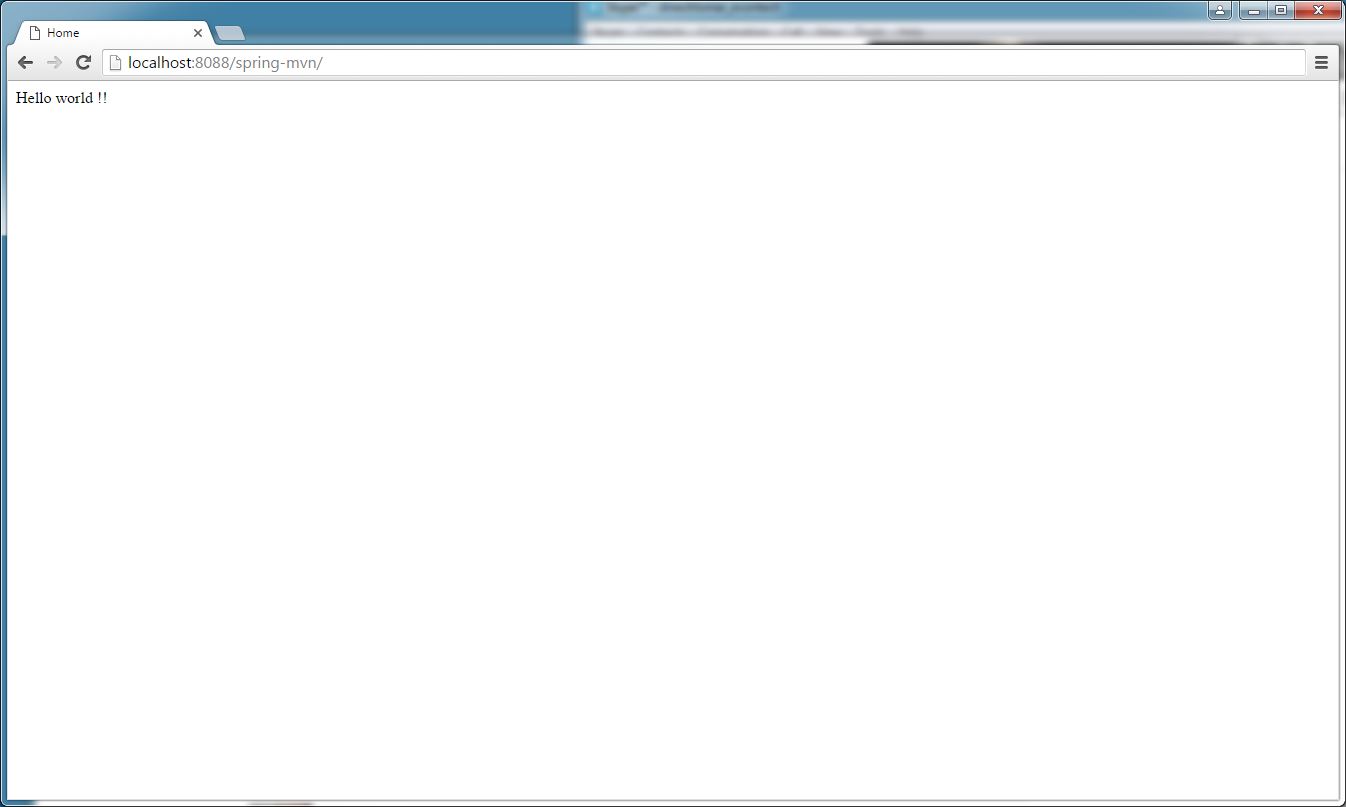
Thanks. Happy coding.
0 Comment(s)